In this tutorial, we’ll use Xcode, Swift, and the Scanbot Credit Card Scanner SDK to create an iOS app for extracting the card number, cardholder name, and expiry date from a credit card.
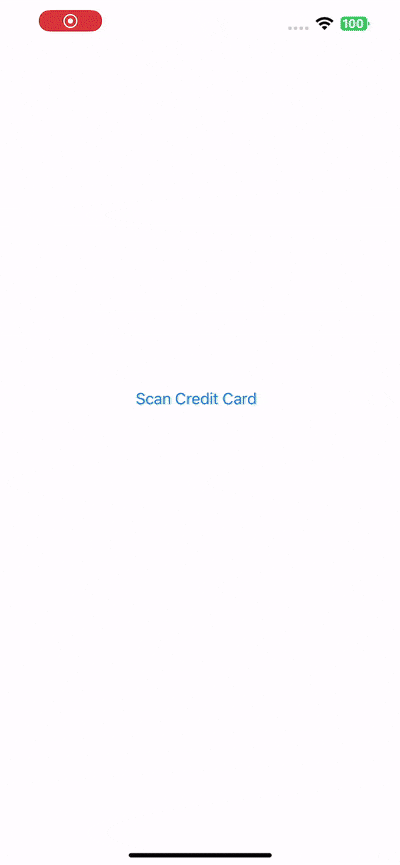
We’ll achieve this in just three easy steps:
- Preparing the project
- Setting up the main screen
- Implementing the credit card scanning feature
All you need is a Mac with the latest version of Xcode and a test device, since we’ll need to use the camera.
Want to see the final code right away? Click here.
ViewController.swift:
import UIKit
import ScanbotSDK
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
}
@IBAction func scanCreditCardButtonTapped(_ sender: Any) {
let configuration = SBSDKUI2CreditCardScannerScreenConfiguration()
SBSDKUI2CreditCardScannerViewController.present(on: self,
configuration: configuration) { result in
if let result {
if let model = result.creditCard?.wrap() as? SBSDKCreditCardDocumentModelCreditCard {
var message = ""
// Retrieve the values:
if let cardNumber = model.cardNumber?.value {
message += "Card number: \(cardNumber.text)\n"
}
if let name = model.cardholderName?.value {
message += "Card holder: \(name.text)\n"
}
if let expiryDate = model.expiryDate?.value {
message += "Expiry date: \(expiryDate.text)\n"
}
let alert = UIAlertController(title: "Result",
message: message,
preferredStyle: .alert)
let action = UIAlertAction(title: "OK",
style: .cancel)
alert.addAction(action)
self.present(alert, animated: true)
}
} else {
let alert = UIAlertController(
title: "No Result",
message: "No credit card data was captured. Please try again.",
preferredStyle: .alert
)
let action = UIAlertAction(title: "OK", style: .cancel)
alert.addAction(action)
self.present(alert, animated: true)
}
}
}
}
Step 1: Prepare the project
Open Xcode and create a new iOS App project. Name the project (e.g., “iOS Credit Card Scanner”), choose Storyboard as the interface, and Swift as the language.
After opening your project, go to File > Add Package Dependencies… and add the Scanbot SDK package for the Swift Package Manager.
Open your Main App Target’s Info tab and add a Privacy – Camera Usage Description key with a value such as “Grant camera access to scan credit cards”.
In AppDelegate.swift, add a line for setting the license key to the application(_:didFinishLaunchingWithOptions:)
method. We don’t need one for this tutorial, but if you have a license key you’d like to use, uncomment the code below and replace <YOUR_LICENSE_KEY>
with your actual key.
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
// Uncomment the following line and replace <YOUR_LICENSE_KEY> with your actual key
// Scanbot.setLicense("<YOUR_LICENSE_KEY>")
return true
}
Without a license key, the SDK will run in trial mode for 60 seconds per session. If you need more than that, you can generate a free trial license.
Step 2: Set up the main screen
We’ll now create a simple UI for starting our Credit Card Scanner.
First, open Main.storyboard and add a UIButton with a meaningful label (e.g., “Scan Credit Card”) to the main view.
After that, create an IBAction for the button in ViewController.swift and implement the button action to present the scanner view controller.
@IBAction func scanCreditCardButtonTapped(_ sender: UIButton) {
// Code to present the scanner view controller will go here
}
We’ll also need to add the following import to ViewController.swift:
import ScanbotSDK
Step 3: Implement the credit card scanning feature
This step involves presenting the Credit Card Scanner view controller. The Scanbot SDK provides a pre-built UI for the scanning process. You’ll need to instantiate the scanner, present it modally, and cast the resulting generic document to the credit card model using the wrap
method.
To do that, add the following code inside scanCreditCardButtonTapped()
in ViewController.swift:
let configuration = SBSDKUI2CreditCardScannerScreenConfiguration()
SBSDKUI2CreditCardScannerViewController.present(on: self,
configuration: configuration) { result in
if let result {
if let model = result.creditCard?.wrap() as? SBSDKCreditCardDocumentModelCreditCard {
var message = ""
// Retrieve the values:
if let cardNumber = model.cardNumber?.value {
message += "Card number: \(cardNumber.text)\n"
}
if let name = model.cardholderName?.value {
message += "Card holder: \(name.text)\n"
}
if let expiryDate = model.expiryDate?.value {
message += "Expiry date: \(expiryDate.text)\n"
}
let alert = UIAlertController(title: "Result",
message: message,
preferredStyle: .alert)
let action = UIAlertAction(title: "OK",
style: .cancel)
alert.addAction(action)
self.present(alert, animated: true)
}
} else {
// Indicates that the cancel button was tapped.
}
}
You can also handle the user canceling the scanning process like this:
} else {
let alert = UIAlertController(
title: "No Result",
message: "No credit card data was captured. Please try again.",
preferredStyle: .alert
)
let action = UIAlertAction(title: "OK", style: .cancel)
alert.addAction(action)
self.present(alert, animated: true)
}
Your final ViewController.swift will then look like this:
import UIKit
import ScanbotSDK
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
}
@IBAction func scanCreditCardButtonTapped(_ sender: Any) {
let configuration = SBSDKUI2CreditCardScannerScreenConfiguration()
SBSDKUI2CreditCardScannerViewController.present(on: self,
configuration: configuration) { result in
if let result {
if let model = result.creditCard?.wrap() as? SBSDKCreditCardDocumentModelCreditCard {
var message = ""
// Retrieve the values:
if let cardNumber = model.cardNumber?.value {
message += "Card number: \(cardNumber.text)\n"
}
if let name = model.cardholderName?.value {
message += "Card holder: \(name.text)\n"
}
if let expiryDate = model.expiryDate?.value {
message += "Expiry date: \(expiryDate.text)\n"
}
let alert = UIAlertController(title: "Result",
message: message,
preferredStyle: .alert)
let action = UIAlertAction(title: "OK",
style: .cancel)
alert.addAction(action)
self.present(alert, animated: true)
}
} else {
let alert = UIAlertController(
title: "No Result",
message: "No credit card data was captured. Please try again.",
preferredStyle: .alert
)
let action = UIAlertAction(title: "OK", style: .cancel)
alert.addAction(action)
self.present(alert, animated: true)
}
}
}
}
Our Credit Card Scanner iOS app is now ready. Build and run it on your test device to read the information on a credit card …
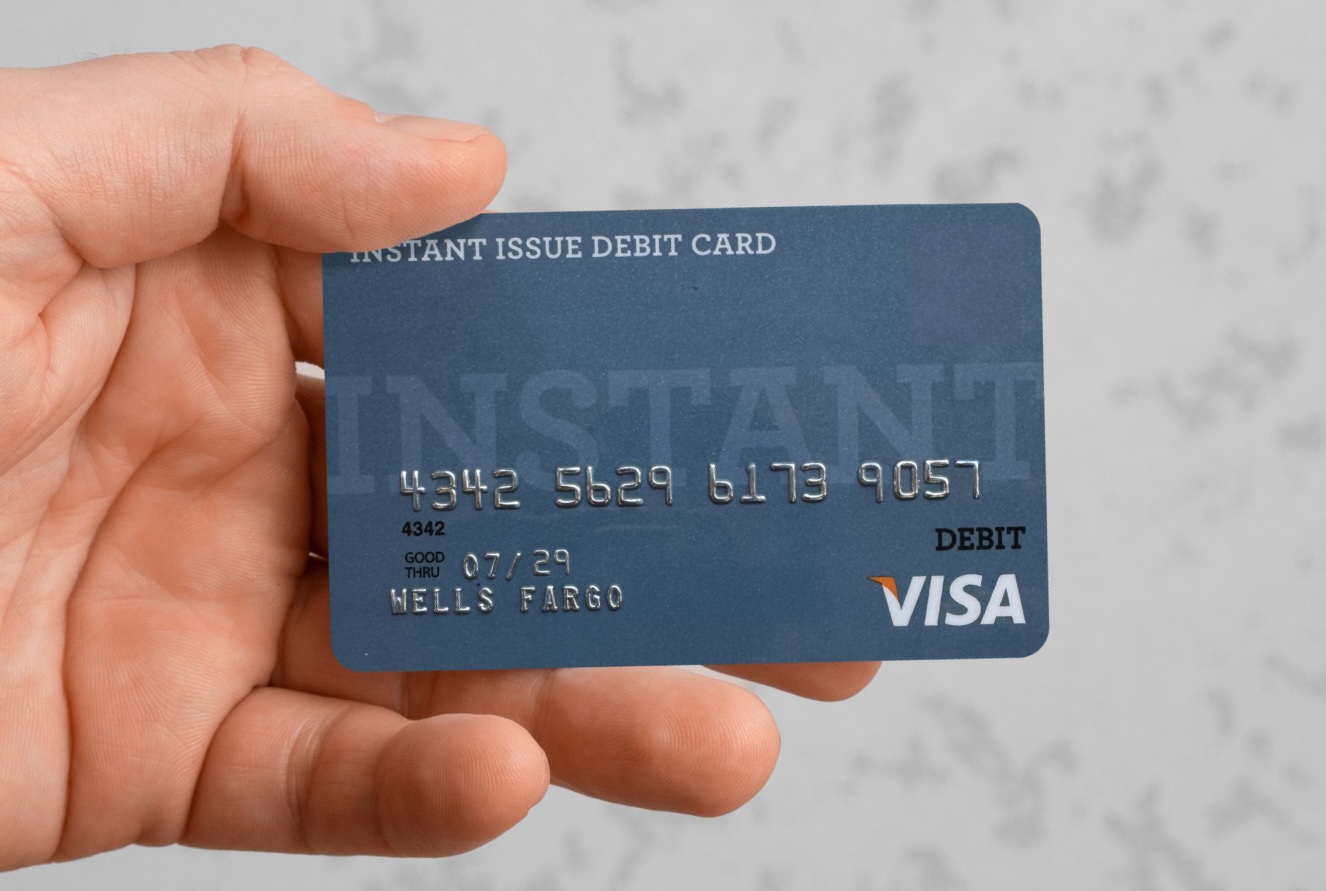
… and test your scanner!
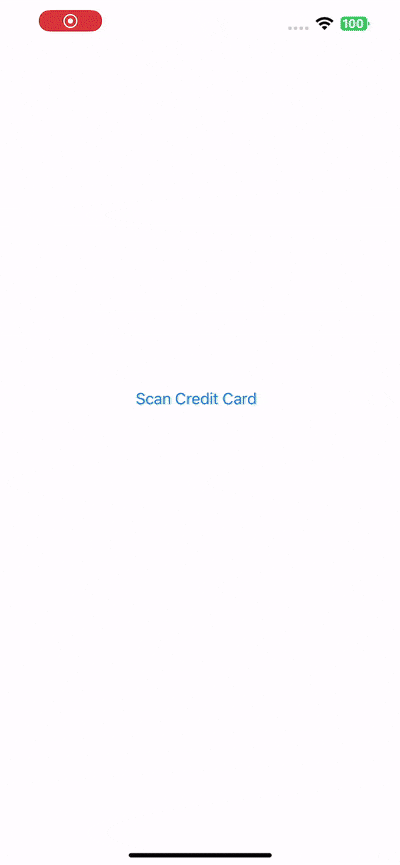
Conclusion
This completes the basic implementation of a Credit Scanner Scanner for iOS using Swift and the Scanbot SDK. We used the SDK’s Ready-to-Use UI Components with their default values for this tutorial, but you can further customize the credit card scanning screen to fit your needs. You can also use the SDK to scan credit cards from image files instead of a live camera stream.
For more information on how to configure the Credit Card Scanner, head over to the documentation.
Should you have questions or run into any issues, we’re happy to help! Just shoot us an email via tutorial-support@scanbot.io.
Happy scanning! 🤳