In this tutorial, we’ll build an Android app for scanning Data Matrix codes using Kotlin, Android Studio, and the Scanbot Android Barcode Scanner SDK.
We’re going to implement two scanning modes that the user can choose from on the start screen. One scans individual Data Matrix codes as soon as they enter the viewfinder and displays their values on the screen. The other uses the SDK’s built-in AR Overlay feature to highlight all detected Data Matrix codes in the viewfinder, enabling the user to tap on each one to add them to a results list.
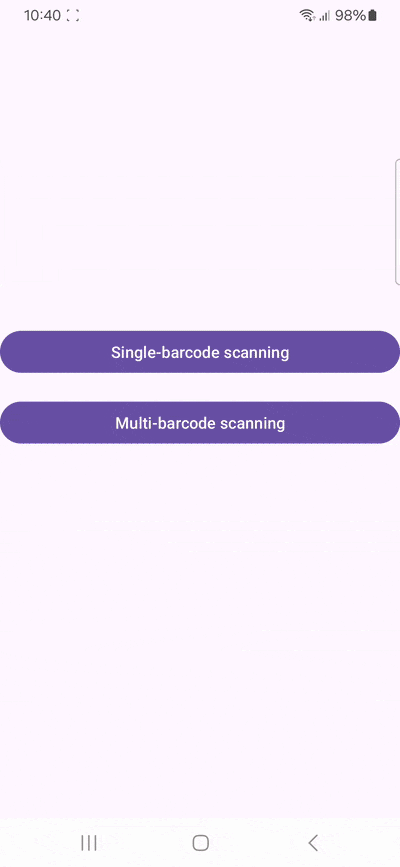
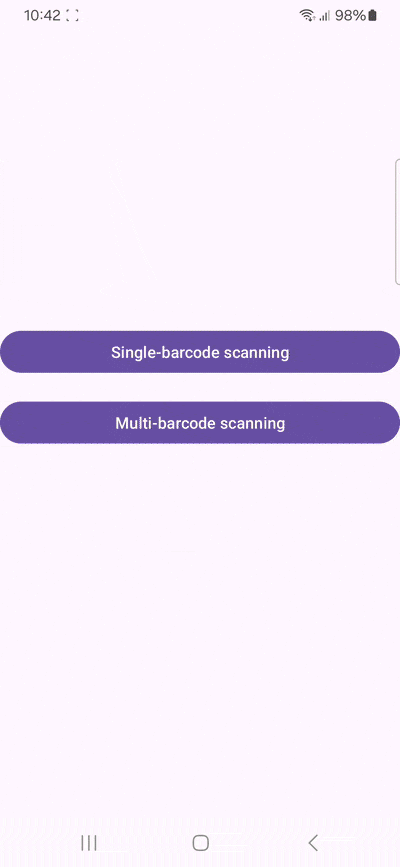
We’ll achieve this in five steps:
- Preparing the project
- Initializing the SDK
- Setting up the main screen
- Implementing the scanning modes
All you need is the latest version of Android Studio and you’re good to go.
Step 1: Prepare the project
Create a new Empty Views Activity and name the project (e.g., “Android Data Matrix Scanner”).
When your project is ready, go to settings.gradle.kts and add the Maven repositories for the Scanbot SDK:
dependencyResolutionManagement {
repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS)
repositories {
google()
mavenCentral()
// Add the repositories here:
maven(url = "https://nexus.scanbot.io/nexus/content/repositories/releases/")
maven(url = "https://nexus.scanbot.io/nexus/content/repositories/snapshots/")
}
}
Now go to app/build.gradle.kts and add the dependencies for the Scanbot SDK and the RTU UI:
dependencies {
implementation("io.scanbot:scanbot-barcode-scanner-sdk:6.2.1")
implementation("io.scanbot:rtu-ui-v2-barcode:6.2.1")
Sync the project.
💡 We use Barcode Scanner SDK version 6.2.1 in this tutorial. You can find the latest version in the changelog.
Since we need to access the device camera to scan Data Matrix codes, let’s also add the necessary permissions in AndroidManifest.xml:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.CAMERA" />
<uses-feature android:name="android.hardware.camera" />
<application
...
Step 2: Initialize the SDK
Before we can use the Barcode Scanner SDK, we need to initialize it. The recommended approach is to do it in your Application
implementation. This ensures the SDK is correctly initialized even when the app’s process is restored after being terminated in the background.
First, we need to create an Application
subclass by right-clicking on the folder app/kotlin+java/com.example.androidbarcodescanner, selecting New > Kotlin Class/File, and naming it (e.g., “ExampleApplication”).
In the resulting ExampleApplication.kt, let’s first add the necessary imports:
import android.app.Application
import io.scanbot.sdk.barcode_scanner.ScanbotBarcodeScannerSDKInitializer
Then make ExampleApplication
extend the Application
class by adding : Application()
and add the code for initializing the SDK inside it:
class ExampleApplication : Application() {
override fun onCreate() {
super.onCreate()
ScanbotBarcodeScannerSDKInitializer()
// Optional: uncomment the next line if you have a license key.
// .license(this, LICENSE_KEY)
.initialize(this)
}
}
💡 Without a license key, our SDK only runs for 60 seconds per session. This is more than enough for the purposes of our tutorial, but if you like, you can generate a license key. Just make sure to change your applicationId
in app/build.gradle.kts to io.scanbot.androidbarcodescanner
and use that ID to generate the license.
Finally, we need to register the ExampleApplication
class in AndroidManifest.xml:
<application
android:name=".ExampleApplication"
...
Step 3: Set up the main screen
We’re going to build a rudimentary UI so we can quickly access the different scanning modes in our finished app.
For this tutorial, we’re going to go with this simple two-button layout, which you can copy into app/res/layout/activity_main.xml:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/btn_single_scanning"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="Single-barcode scanning"
app:layout_constraintBottom_toTopOf="@id/btn_multi_scanning"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
android:layout_marginBottom="20dp" />
<Button
android:id="@+id/btn_multi_scanning"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="Multi-barcode scanning"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
android:layout_marginTop="20dp"
android:layout_marginBottom="20dp" />
</androidx.constraintlayout.widget.ConstraintLayout>
Step 4: Implement the scanning modes
Now we’ll connect each button with one of our RTU UI’s scanning modes, starting with single-barcode scanning.
Go to MainActivity.kt and add the necessary imports:
import android.widget.Button
import android.widget.Toast
import androidx.activity.result.ActivityResultLauncher
import io.scanbot.sdk.ui_v2.barcode.BarcodeScannerActivity
import io.scanbot.sdk.ui_v2.common.activity.registerForActivityResultOk
import io.scanbot.sdk.ui_v2.barcode.configuration.*
With that out of the way, let’s implement the first scanning mode.
Single-barcode scanning
In the MainActivity
class, add a private property that we’ll use to launch the barcode scanner.
private val barcodeResultLauncher: ActivityResultLauncher<BarcodeScannerConfiguration> =
registerForActivityResultOk(BarcodeScannerActivity.ResultContract()) { resultEntity ->
// Barcode Scanner result callback:
// Get the first scanned barcode from the result object...
val barcodeItem = resultEntity.result?.items?.first()
// ... and process the result as needed. For example, display as a Toast:
Toast.makeText(
this,
"Scanned: ${barcodeItem?.text} (${barcodeItem?.type})",
Toast.LENGTH_LONG
).show()
}
Next, in the onCreate()
method, we set up an OnClickListener
for our Single-barcode scanning button. When the button is clicked, it should launch barcodeResultLauncher
using a configuration object we’ll set up in the next step:
findViewById<Button>(R.id.btn_single_scanning).setOnClickListener {
barcodeResultLauncher.launch(config)
}
Now paste the configuration for single-barcode scanning above barcodeResultLauncher.launch(config)
:
findViewById<Button>(R.id.btn_single_scanning).setOnClickListener {
val config = BarcodeScannerConfiguration().apply {
// Initialize the use case for single scanning.
this.useCase = SingleScanningMode().apply {
// Enable and configure the confirmation sheet.
this.confirmationSheetEnabled = true
}
// Restrict scanning to Data Matrix codes
this.recognizerConfiguration.barcodeFormats = arrayOf(BarcodeFormat.DATA_MATRIX).toList()
}
barcodeResultLauncher.launch(config)
}
💡 Restricting scanning to Data Matrix codes prevents unintended scans and improves the scanner’s performance, as it doesn’t need to check each supported symbology.
Feel free to run the app and try the single-barcode scanning feature before we move on to the next mode.
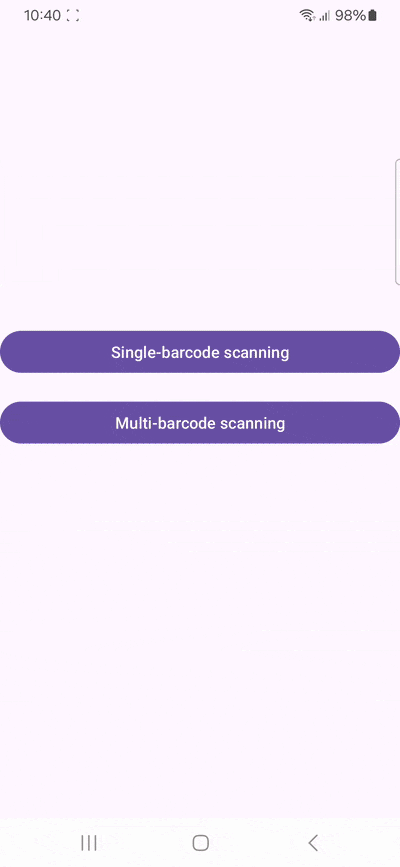
Multi-barcode scanning with AR Overlay
Set up another OnClickListener
, this time for the Multi-barcode scanning button …
findViewById<Button>(R.id.btn_multi_scanning).setOnClickListener {
barcodeResultLauncher.launch(config)
}
… and paste in the configuration for multi-barcode scanning above barcodeResultLauncher.launch(config)
:
findViewById<Button>(R.id.btn_multi_scanning).setOnClickListener {
val config = BarcodeScannerConfiguration().apply {
// Initialize the use case for multiple scanning.
this.useCase = MultipleScanningMode().apply {
// Set the counting mode.
this.mode = MultipleBarcodesScanningMode.COUNTING
// Set the sheet mode for the barcodes preview.
this.sheet.mode = SheetMode.COLLAPSED_SHEET
// Set the height for the collapsed sheet.
this.sheet.collapsedVisibleHeight = CollapsedVisibleHeight.LARGE
// Enable manual count change.
this.sheetContent.manualCountChangeEnabled = true
// Configure the AR Overlay.
this.arOverlay.visible = true
this.arOverlay.automaticSelectionEnabled = false
}
// Set an array of accepted barcode types.
this.recognizerConfiguration.barcodeFormats = arrayOf(BarcodeFormat.DATA_MATRIX).toList()
}
barcodeResultLauncher.launch(config)
}
Build and run the app.
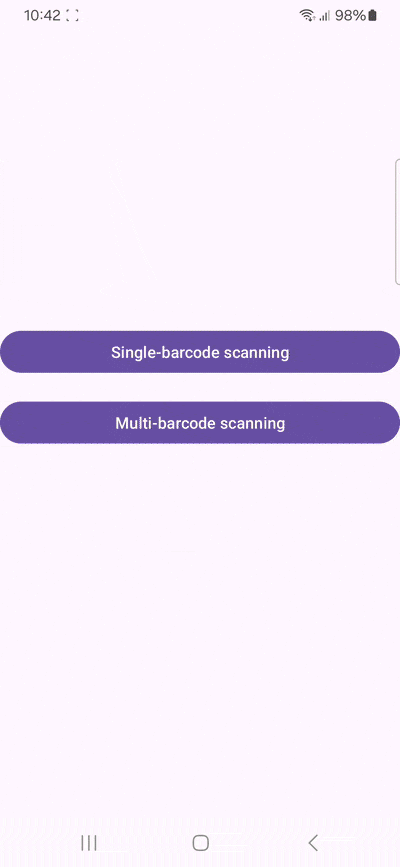
Conclusion
🎉 Congratulations! You can now scan Data Matrix codes one at a time or preview them with the AR Overlay before adding them to the results list.
If this tutorial has piqued your interest in integrating barcode scanning functionalities into your Android app, make sure to take a look at our SDK’s other neat features in our documentation – or run our example project for a more hands-on experience.
Should you have questions about this tutorial or run into any issues, we’re happy to help! Just shoot us an email via tutorial-support@scanbot.io.
Happy scanning! 🤳