Setting up an app in .NET MAUI capable of scanning any 1D or 2D barcode is very straightforward. All we need are the following steps:
- Prepare the project
- Install the SDK
- Initialize the SDK
- Implement the scanning modules
- Scan some barcodes!
Thanks to our SDK’s Ready-to-Use UI Components, you can even use an AR overlay to display multiple barcodes’ contents right in the viewfinder.
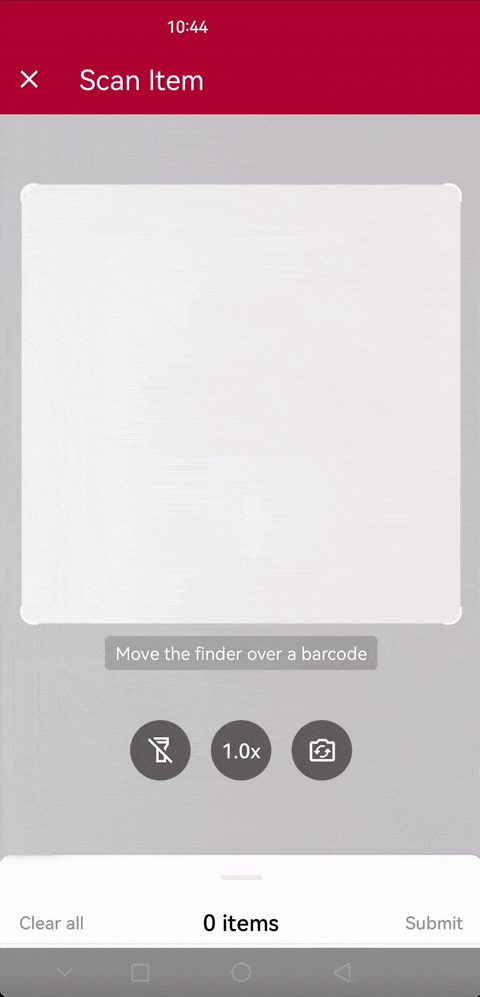
Requirements
There are several options for developing .NET MAUI applications, including the ability to build and launch projects from the .NET CLI if you are so inclined. Whatever method you choose, make sure to download the latest .NET version for your development platform of choice. You’ll also need Microsoft’s build of OpenJDK 17.
In this tutorial, we’re going to develop our app with Android and iOS as target platforms.
⚠️ A note for Windows users: If you want to develop your app for iOS, additional setup is required, including having a Mac available as a build server. You can learn more about this in the .NET MAUI documentation. In this tutorial, we’ll assume you’re developing on a Mac and using the CLI.
If this is your first time developing a .NET MAUI application on your machine, execute the following command:
sudo dotnet workload install maui
1. Preparing the project
First, create a new directory for your project and navigate into it:
mkdir test-maui
cd test-maui
Then create the project with:
dotnet new maui
Since we need access to the device cameras to scan barcodes, let’s add the necessary camera permissions for Android and iOS.
For Android, add the following to Platforms/Android/AndroidManifest.xml inside the <manifest>
element:
<uses-permission android:name="android.permission.CAMERA" />
<uses-feature android:name="android.hardware.camera" />
For iOS, add the following to Platforms/iOS/Info.plist anywhere inside the <dict>
element:
<key>NSCameraUsageDescription</key>
<string>Please provide camera access.</string>
2. Installing the SDK
In this tutorial, we’re using SDK version 6.1.0. You can find the latest version in our changelog.
Add the following code to the test-maui.csproj file in your project folder:
<ItemGroup>
<PackageReference Condition="$(TargetFramework.Contains('ios'))" Include="ScanbotBarcodeSDK.MAUI" Version="6.1.0" />
<PackageReference Condition="$(TargetFramework.Contains('android'))" Include="ScanbotBarcodeSDK.MAUI" Version="6.1.0" />
</ItemGroup>
Then run dotnet restore
.
💡 If there are package conflicts, add the appropriate <PackageReference>
tags to the project and make sure <PackageReference>
has NoWarn="NU1605"
added to it to suppress the related build error for that particular package.
Now that the project is set up, we can start integrating the barcode scanning functionalities.
3. Initializing the SDK
In MauiProgram.cs, initialize the Barcode Scanner SDK by replacing the contents with the following code:
using Microsoft.Extensions.Logging;
using ScanbotSDK.MAUI;
// Replace test_maui with the namespace of your app.
namespace test_maui;
public static class MauiProgram
{
// Without a license key, the Scanbot Barcode SDK will work for 1 minute.
// To scan longer, register for a trial license key here: https://scanbot.io/trial/
public const string LicenseKey = "";
public static MauiApp CreateMauiApp()
{
var builder = MauiApp.CreateBuilder();
builder
.UseMauiApp<App>()
.ConfigureFonts(fonts =>
{
fonts.AddFont("OpenSans-Regular.ttf", "OpenSansRegular");
fonts.AddFont("OpenSans-Semibold.ttf", "OpenSansSemibold");
});
#if DEBUG
builder.Logging.AddDebug();
#endif
SBSDKInitializer.Initialize(LicenseKey, new SBSDKConfiguration
{
EnableLogging = true,
ErrorHandler = (status, feature) =>
{
Console.WriteLine($"License error: {status}, {feature}");
}
});
return builder.Build();
}
}
💡 Without a license key, our SDK only runs for 60 seconds per session. This is more than enough for the purposes of our tutorial, but if you like, you can generate a license key using the <ApplicationId>
found in test-maui.csproj.
For the scanning components to work correctly in Android, you also need to add DependencyManager.RegisterActivity(this)
to the OnCreate
method in Platforms/Android/MainActivity.cs:
protected override void OnCreate(Bundle? savedInstanceState)
{
base.OnCreate(savedInstanceState);
ScanbotSDK.MAUI.DependencyManager.RegisterActivity(this);
}
💡 If there’s no OnCreate
method in your MainActivity.cs, add the above code snippet to the MainActivity
class.
4. Implementing the scanning modes
Our RTU UI components make it easy to deploy our Barcode Scanner SDK’s different scanning modes in your app. Let’s start with the simplest use case: single-barcode scanning.
In your project folder, go to MainPage.xaml.cs and replace the content with the following code:
using ScanbotSDK.MAUI;
using ScanbotSDK.MAUI.Barcode;
using ScanbotSDK.MAUI.Common;
// Replace test_maui with the namespace of your app.
namespace test_maui;
public partial class MainPage : ContentPage
{
int count = 0;
public MainPage()
{
InitializeComponent();
}
private async void StartSingleScanning(object sender, EventArgs e)
{
try
{
if (!ScanbotSDKMain.LicenseInfo.IsValid)
{
await DisplayAlert(title: "License invalid", message: "Trial license expired.", cancel: "Dismiss");
return;
}
// Create the default configuration object.
var configuration = new BarcodeScannerConfiguration();
// Initialize the single-scan use case.
var singleUsecase = new SingleScanningMode();
// Enable and configure the confirmation sheet.
singleUsecase.ConfirmationSheetEnabled = true;
singleUsecase.SheetColor = Color.FromArgb("#FFFFFF");
// Hide/unhide the barcode image of the confirmation sheet.
singleUsecase.BarcodeImageVisible = true;
// Configure the barcode title of the confirmation sheet.
singleUsecase.BarcodeTitle.Visible = true;
singleUsecase.BarcodeTitle.Color = Color.FromArgb("#000000");
// Configure the barcode subtitle of the confirmation sheet.
singleUsecase.BarcodeSubtitle.Visible = true;
singleUsecase.BarcodeSubtitle.Color = Color.FromArgb("#000000");
// Configure the cancel button of the confirmation sheet.
singleUsecase.CancelButton.Text = "Close";
singleUsecase.CancelButton.Foreground.Color = Color.FromArgb("#C8193C");
singleUsecase.CancelButton.Background.FillColor = Color.FromArgb("#00000000");
// Configure the submit button of the confirmation sheet.
singleUsecase.SubmitButton.Text = "Submit";
singleUsecase.SubmitButton.Foreground.Color = Color.FromArgb("#FFFFFF");
singleUsecase.SubmitButton.Background.FillColor = Color.FromArgb( "#C8193C");
// Set the configured use case.
configuration.UseCase = singleUsecase;
// Create and set an array of accepted barcode formats.
configuration.RecognizerConfiguration.BarcodeFormats = BarcodeFormats.Common;
var result = await ScanbotSDKMain.RTU.BarcodeScanner.LaunchAsync(configuration);
var barcodeAsText = result.Items.Select(barcode => $"{barcode.Type}: {barcode.Text}")
.FirstOrDefault() ?? string.Empty;
await DisplayAlert("Found barcode", barcodeAsText, "Finish");
}
catch (TaskCanceledException)
{
// For when the user cancels the action.
}
catch (Exception ex)
{
// For any other errors that occur.
Console.WriteLine(ex.Message);
}
}
}
Now let’s go to MainPage.xaml and edit the button on the start screen so it calls our StartSingleScanning
method when clicked:
<Button
x:Name="SingleScanningBtn"
Text="Start single-barcode scanning"
SemanticProperties.Hint="Starts the process to scan a single barcode"
Clicked="StartSingleScanning"
HorizontalOptions="Fill" />
If you like, you can build and run the app to see if everything is working correctly so far:
iOS: dotnet build . -f net8.0-ios -t:Run -r ios-arm64
Android: dotnet build . -f net8.0-android -t:Run
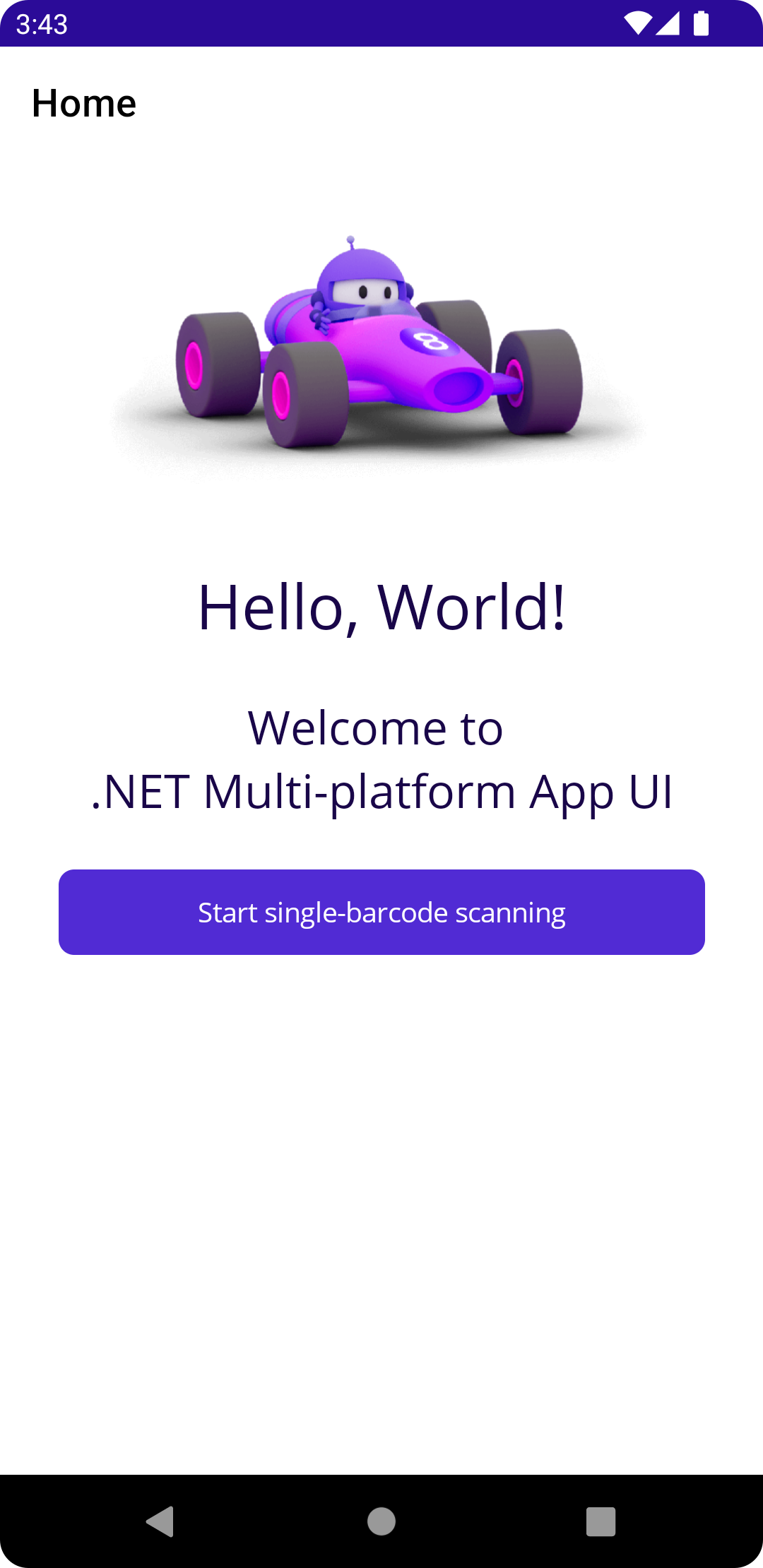
In the next step, we’re going to insert both the multi-barcode scanning and the AR overlay modules.
Let’s go back to MainPage.xaml.cs and insert the two methods StartMultiScanning
and StartAROverlay
:
private async void StartMultiScanning(object sender, EventArgs e)
{
try
{
if (!ScanbotSDKMain.LicenseInfo.IsValid)
{
await DisplayAlert(title: "License invalid", message: "Trial license expired.", cancel: "Dismiss");
return;
}
// Create the default configuration object.
var configuration = new BarcodeScannerConfiguration();
// Initialize the multi-scan use case.
var multiUsecase = new MultipleScanningMode();
// Set the counting repeat delay.
multiUsecase.CountingRepeatDelay = 1000;
// Set the counting mode.
multiUsecase.Mode = MultipleBarcodesScanningMode.Counting;
// Set the sheet mode of the barcodes preview.
multiUsecase.Sheet.Mode = SheetMode.CollapsedSheet;
// Set the height of the collapsed sheet.
multiUsecase.Sheet.CollapsedVisibleHeight = CollapsedVisibleHeight.Large;
// Enable manual count change.
multiUsecase.SheetContent.ManualCountChangeEnabled = true;
// Configure the submit button.
multiUsecase.SheetContent.SubmitButton.Text = "Submit";
multiUsecase.SheetContent.SubmitButton.Foreground.Color = Color.FromArgb("#000000");
configuration.UseCase = multiUsecase;
// Create and set an array of accepted barcode formats.
configuration.RecognizerConfiguration.BarcodeFormats = BarcodeFormats.Common;
var result = await ScanbotSDKMain.RTU.BarcodeScanner.LaunchAsync(configuration);
var barcodesAsText = result.Items.Select(barcode => $"{barcode.Type}: {barcode.Text}").ToArray();
await DisplayActionSheet("Found barcodes", "Finish", null, barcodesAsText);
}
catch (TaskCanceledException)
{
// For when the user cancels the action.
}
catch (Exception ex)
{
// For any other errors that occur.
Console.WriteLine(ex.Message);
}
}
private async void StartAROverlay(object sender, EventArgs e)
{
try
{
if (!ScanbotSDKMain.LicenseInfo.IsValid)
{
await DisplayAlert(title: "License invalid", message: "Trial license expired.", cancel: "Dismiss");
return;
}
// Create the default configuration object.
var configuration = new BarcodeScannerConfiguration();
// Configure the usecase.
var usecase = new MultipleScanningMode();
usecase.Mode = MultipleBarcodesScanningMode.Unique;
usecase.Sheet.Mode = SheetMode.CollapsedSheet;
usecase.Sheet.CollapsedVisibleHeight = CollapsedVisibleHeight.Small;
// Configure AR Overlay.
usecase.ArOverlay.Visible = true;
usecase.ArOverlay.AutomaticSelectionEnabled = false;
// Set the configured usecase.
configuration.UseCase = usecase;
// Create and set an array of accepted barcode formats.
configuration.RecognizerConfiguration.BarcodeFormats = BarcodeFormats.Common;
var result = await ScanbotSDKMain.RTU.BarcodeScanner.LaunchAsync(configuration);
var barcodeAsText = result.Items.Select(barcode => $"{barcode.Type}: {barcode.Text}")
.FirstOrDefault() ?? string.Empty;
await DisplayAlert("Found barcode", barcodeAsText, "Finish");
}
catch (TaskCanceledException)
{
// For when the user cancels the action.
}
catch (Exception ex)
{
// For any other errors that occur.
Console.WriteLine(ex.Message);
}
}
And let’s not forget to insert the buttons in our MainPage.xaml:
<Button
x:Name="MultiScanningBtn"
Text="Start multi-barcode scanning"
SemanticProperties.Hint="Starts the process to scan multiple barcodes"
Clicked="StartMultiScanning"
HorizontalOptions="Fill" />
<Button
x:Name="AROverlayBtn"
Text="Starts the AR overlay"
SemanticProperties.Hint="Starts the process to scan barcodes using an AR overlay"
Clicked="StartAROverlay"
HorizontalOptions="Fill" />
Now let’s build and run the app again so we can try out our new scanning modules.
💡 If you run into errors while building the Android app, try the following command:
dotnet build . -f net8.0-android -t:Run --no-incremental
The option --no-incremental
disables incremental building. When used, it forces a clean rebuild of the entire project, ignoring any previously built components.
5. Scanning some barcodes!
Now you can go ahead and scan 1D and 2D barcodes – one after the other, many at the same time, and even with an AR overlay that lets you preview their values!
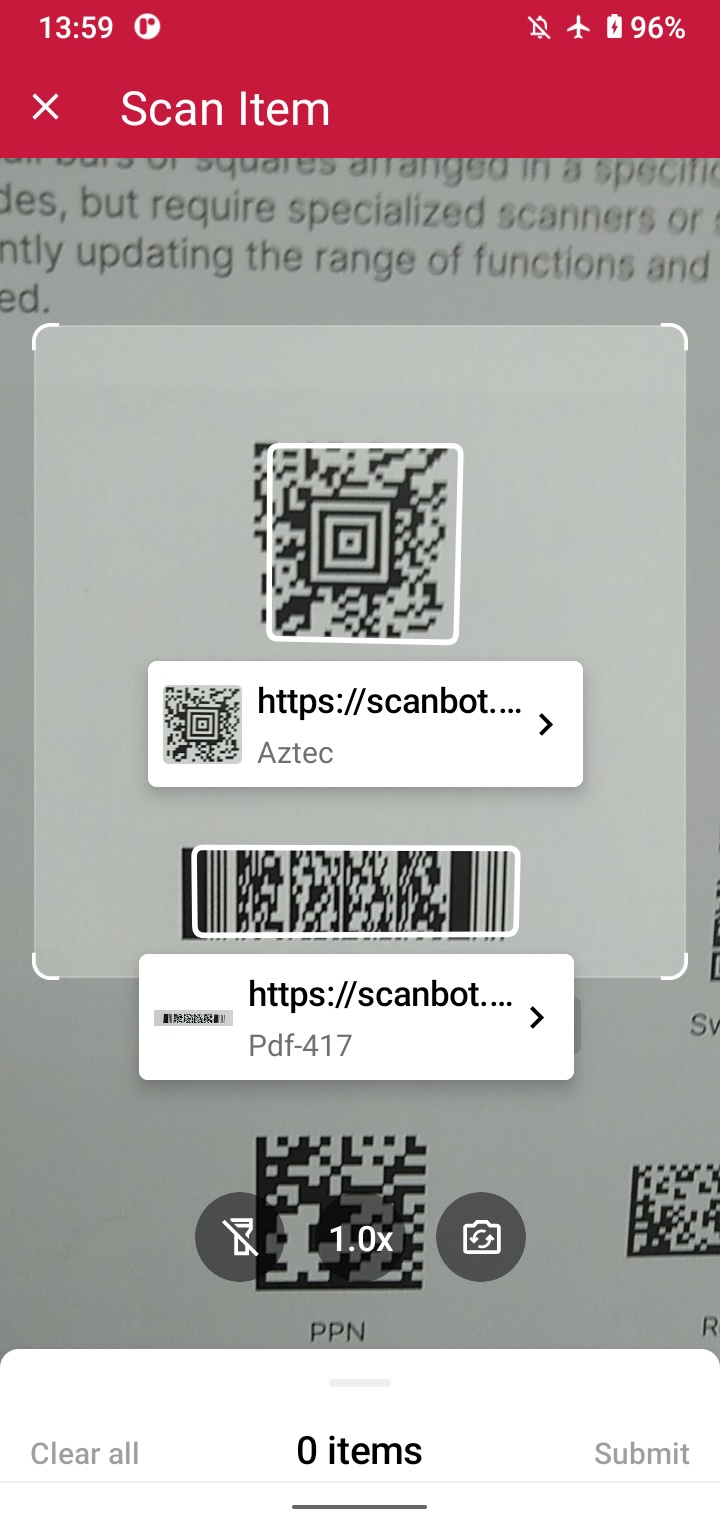
And if you’re in need of some sample barcodes for testing purposes, we’ve got you covered:
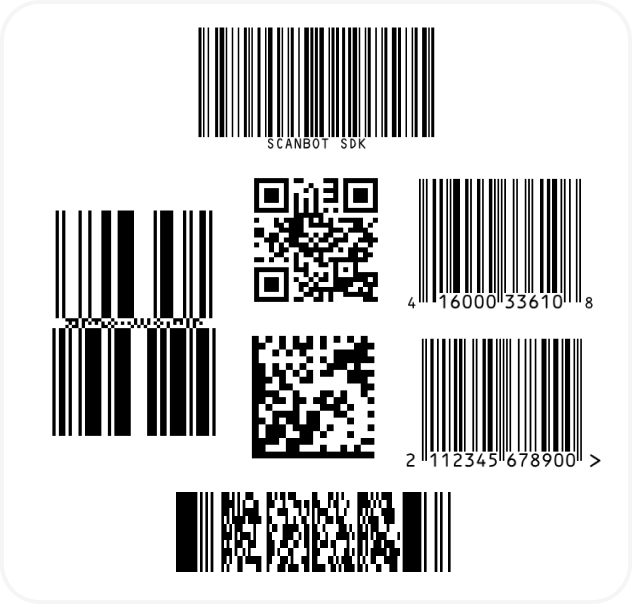
If this tutorial has piqued your interest in integrating barcode scanning functionalities into your MAUI app, make sure to take a look at our SDK’s other neat features in our documentation – or run our example project for a more hands-on experience!
Should you have questions about this tutorial or ran into any issues, we’re happy to help! Just shoot us an email via tutorial-support@scanbot.io.
Happy coding!