Introduction
Setting up a simple app that can scan any 1D or 2D barcode is very straightforward – all we need are the following steps:
- Create a project
- Install the SDK
- Set up the main screen
- Implement the scanning modes
- Build, run, and scan!
After that, you will be scanning barcodes with lightning speed! ⚡
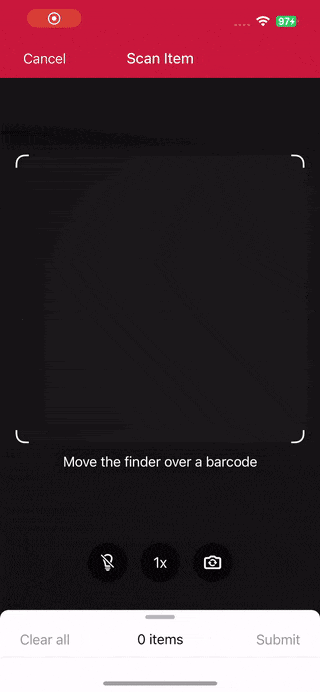
Requirements
All you need for this project is the following:
- macOS with Xcode 14.0 or higher
- A test device, since we’ll need to use the camera (minimum deployment target: iOS 13.0)
- Basic knowledge of how to build an iOS app
💡 Our iOS Barcode Scanner SDK supports both Swift and Objective-C, but we’ll stick to Swift for this tutorial.
Preparing the project
First, we’re going to create a new Swift project with the Storyboard interface.
To create the project:
- Open Xcode and click on Create New Project… (or go to File -> New -> Project…)
- Make sure you have the iOS tab selected, then choose App.
- Give it a name, e.g., MyBarcodeScanningApp.
- Choose Storyboard as the interface.
- Select Swift as the programming language.
Next, we’ll install the Barcode Scanner SDK package.
Installing the SDK
For this tutorial, we will use the Swift Package Manager to install the Barcode Scanner SDK.
- In Xcode, go to File -> Add Package Dependencies…
- Paste the following package URL into the search bar: https://github.com/doo/scanbot-barcode-scanner-sdk-ios-spm.git
- Select the package and click Add Package.
- In the pop-up that appears after verification, for “Add to Target”, choose your project.
- Click Add Package again.
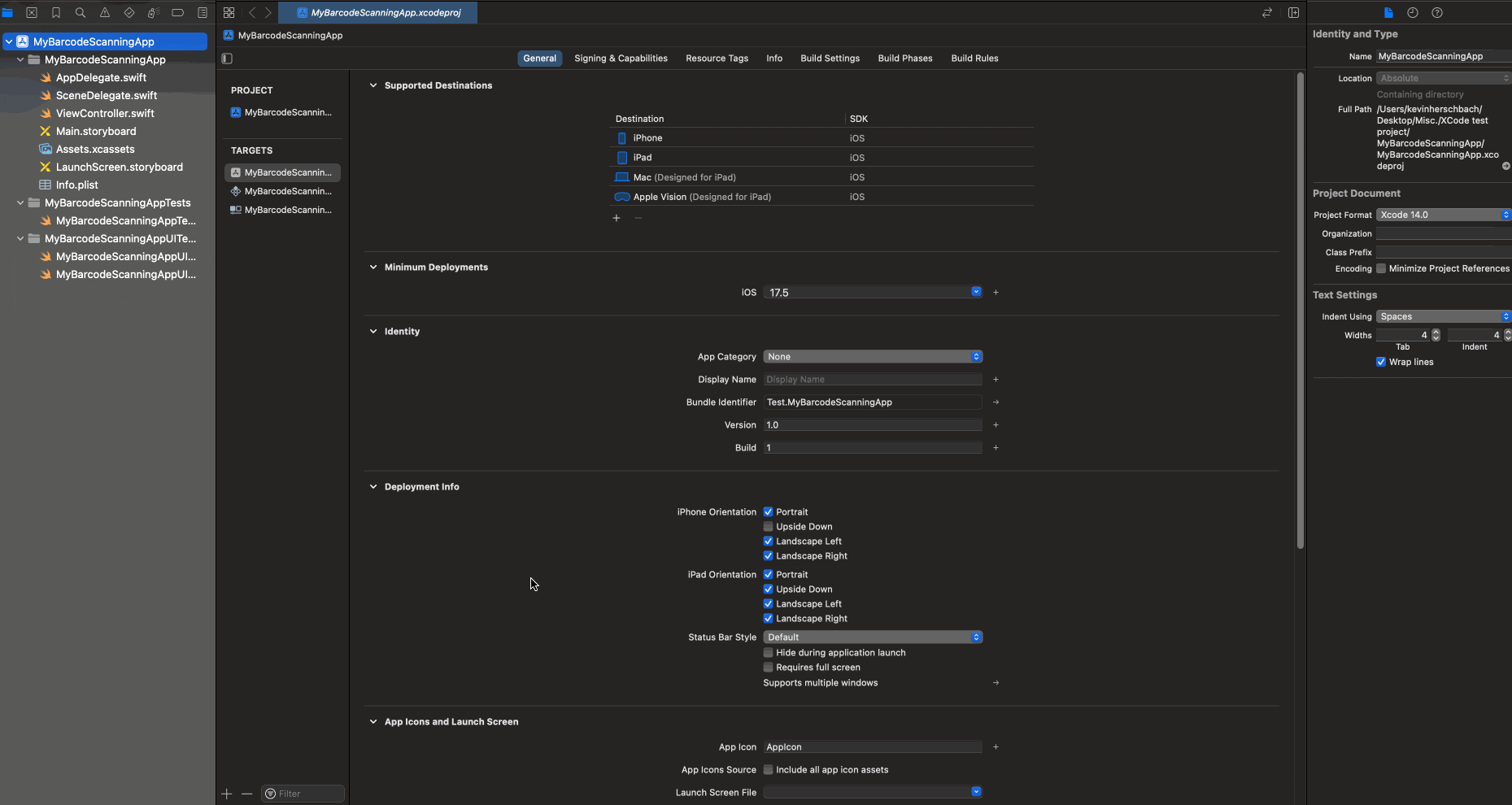
💡 Without a license key, our SDK only runs for 60 seconds per session. This is more than enough for the purposes of our tutorial, but if you like, you can generate a license key here.
You can activate your license in the code by using the +[Scanbot setLicense:]
method of the Scanbot
class. Execute this code as early as possible during your app’s runtime and before using any Scanbot SDK functionality, e.g., in your application class (AppDelegate
).
If your license has expired, invocations of the Scanbot Barcode SDK API will not work. You can check for license expiration during runtime by using the +[Scanbot isLicenseValid]
method.
For more information about license checks in your app, please refer to our documentation.
We’ll need access to the device camera to scan barcodes, so let’s set the corresponding privacy property now.
In the project navigator, select your project, then select your app under “TARGETS”. Open the tab Info, add the target property Privacy – Camera Usage Description, and type in a meaningful value, e.g., “Grant access to your camera to start scanning”.
Integrating the scanning functionalities
Now that the project is set up, integrating barcode scanning into your app is very straightforward.
In fact, it’s so straightforward that we’ll implement not just one, but three different scanning modes in our project:
- Single-barcode scanning: Read and show the value of a single barcode.
- Multi-barcode scanning: Read as many barcodes as you like and display their values in a list.
- AR overlay: Display the values of the barcodes in the viewfinder on the screen in real time.
Here’s how we’re going to achieve that:
- Step 1: Set up the main screen (create three buttons and connect them to our View Controller)
- Step 2: Implement the scanning modes (copy and paste the code for the three scanning modes into the
@IBAction
functions) - Step 2.5 (optional): Customize the scanning UI
- Step 3: Start scanning 🤳
Let’s dive right in!
Step 1: Setting up the main screen
How exactly you approach this is up to you, but for the sake of this tutorial, we will just add three UIButton
elements (“Single-Barcode Scanning”, “Multi-Barcode Scanning”, and “AR Overlay”) onto the View Controller Scene in Main.storyboard. Then, we connect them as an Action to ViewController.swift by dragging them while holding the control key.
For our purposes, let’s call the resulting @IBAction
functions “startSingleScanning”, “startMultiScanning”, and “startAROverlay”.
We also need to import the ScanbotBarcodeScannerSDK package like so:
import ScanbotBarcodeScannerSDK
In the next step, we’ll add the code to get our scanning interface up and running into those functions.
Step 2: Implementing the scanning modes
Thanks to our RTU UI components, integrating our Barcode Scanner SDK’s features into your app is a breeze!
In fact, you can just copy and paste the code from our documentation 🦥
Here’s the code to put in each function for your convenience:
For single-barcode scanning:
...
@IBAction func startSingleScanning(_ sender: Any) {
// Create the default configuration object.
let configuration = SBSDKUI2BarcodeScannerConfiguration()
// Initialize the single-scan use case.
let singleUsecase = SBSDKUI2SingleScanningMode()
// Enable and configure the confirmation sheet.
singleUsecase.confirmationSheetEnabled = true
singleUsecase.sheetColor = SBSDKUI2Color(colorString: "#FFFFFF")
// Hide/unhide the barcode image of the confirmation sheet.
singleUsecase.barcodeImageVisible = true
// Configure the barcode title of the confirmation sheet.
singleUsecase.barcodeTitle.visible = true
singleUsecase.barcodeTitle.color = SBSDKUI2Color(colorString: "#000000")
// Configure the barcode subtitle of the confirmation sheet.
singleUsecase.barcodeSubtitle.visible = true
singleUsecase.barcodeSubtitle.color = SBSDKUI2Color(colorString: "#000000")
// Configure the cancel button of the confirmation sheet.
singleUsecase.cancelButton.text = "Close"
singleUsecase.cancelButton.foreground.color = SBSDKUI2Color(colorString: "#C8193C")
singleUsecase.cancelButton.background.fillColor = SBSDKUI2Color(colorString: "#00000000")
// Configure the submit button of the confirmation sheet.
singleUsecase.submitButton.text = "Submit"
singleUsecase.submitButton.foreground.color = SBSDKUI2Color(colorString: "#FFFFFF")
singleUsecase.submitButton.background.fillColor = SBSDKUI2Color(colorString: "#C8193C")
// Set the configured use case.
configuration.useCase = singleUsecase
// Create and set an array of accepted barcode formats.
configuration.recognizerConfiguration.barcodeFormats = SBSDKUI2BarcodeFormat.twoDFormats
// Present the recognizer view controller modally on this view controller.
SBSDKUI2BarcodeScannerViewController.present(on: self,
configuration: configuration) { controller, cancelled, error, result in
// Completion handler to process the result.
// The `cancelled` parameter indicates whether the cancel button was tapped.
controller.presentingViewController?.dismiss(animated: true)
}
...
For multi-barcode scanning:
...
@IBAction func startMultiScanning(_ sender: Any) {
// Create the default configuration object.
let configuration = SBSDKUI2BarcodeScannerConfiguration()
// Initialize the multi-scan use case.
let multiUsecase = SBSDKUI2MultipleScanningMode()
// Set the counting repeat delay.
multiUsecase.countingRepeatDelay = 1000
// Set the counting mode.
multiUsecase.mode = .counting
// Set the sheet mode of the barcodes preview.
multiUsecase.sheet.mode = .collapsedSheet
// Set the height of the collapsed sheet.
multiUsecase.sheet.collapsedVisibleHeight = .large
// Enable manual count change.
multiUsecase.sheetContent.manualCountChangeEnabled = true
// Configure the submit button.
multiUsecase.sheetContent.submitButton.text = "Submit"
multiUsecase.sheetContent.submitButton.foreground.color = SBSDKUI2Color(colorString: "#000000")
// Set the configured use case.
configuration.useCase = multiUsecase
// Create and set an array of accepted barcode formats.
configuration.recognizerConfiguration.barcodeFormats = SBSDKUI2BarcodeFormat.twoDFormats
// Present the recognizer view controller modally on this view controller.
SBSDKUI2BarcodeScannerViewController.present(on: self,
configuration: configuration) { controller, cancelled, error, result in
// Completion handler to process the result.
// The `cancelled` parameter indicates whether the cancel button was tapped.
controller.presentingViewController?.dismiss(animated: true)
}
...
For the AR overlay:
...
@IBAction func startAROverlay(_ sender: Any) {
// Create the default configuration ject.
let configuration = SBSDKUI2BarcodeScannerConfiguration()
// Configure the usecase.
let usecase = SBSDKUI2MultipleScanningMode()
usecase.mode = .unique
usecase.sheet.mode = .collapsedSheet
usecase.sheet.collapsedVisibleHeight = .small
// Configure AR Overlay.
usecase.arOverlay.visible = true
usecase.arOverlay.automaticSelectionEnabled = false
// Set the configured usecase.
configuration.useCase = usecase
// Create and set an array of accepted barcode formats.
configuration.recognizerConfiguration.barcodeFormats = SBSDKUI2BarcodeFormat.twoDFormats
// Present the recognizer view controller modally on this view controller.
SBSDKUI2BarcodeScannerViewController.present(on: self,
configuration: configuration) { controller, cancelled, error, result in
// Completion handler to process the result.
// The `cancelled` parameter indicates whether the cancel button was tapped.
controller.presentingViewController?.dismiss(animated: true)
}
}
}
There’s one tiny thing we’ll change here right away, though: We want to be able to scan both 1D and 2D barcode formats.
For this, let’s go to configuration.recognizerConfiguration.barcodeFormats
in each @IBAction
function and change SBSDKUI2BarcodeFormat.twoDFormats
to SBSDKUI2BarcodeFormat.commonFormats
.
Let’s now explore some of the other customization options.
Step 2.5 (optional): Customizing the scanning UI
Our RTU UI not only makes it very easy to integrate our SDK’s scanning functionalities, but also to style the UI elements in any way you like 🎨
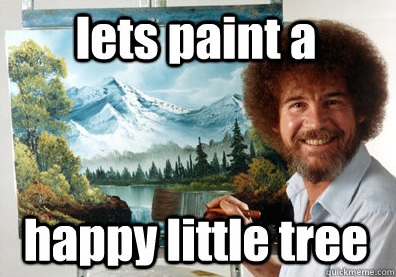
The comments already give you a hint about what’s possible to change:
- Hide or show specific UI elements
- Configure the active and inactive states of some UI elements
- Change colors
- Change titles and subtitles
- Change the accepted barcode formats
- Control the barcode sheet behavior for multi-scanning
- … and more
As an example, let’s take a closer look at some of the configuration options of our multi-barcode scanning feature:
// Set the counting mode.
multiUsecase.mode = .counting
Here, we can change .counting
to .unique
. This will prevent the SDK from scanning multiple barcodes with the same value, which is helpful if you only want to count the number of unique barcodes.
// Set the sheet mode of the barcodes preview.
multiUsecase.sheet.mode = .collapsedSheet
Change .collapsedSheet
to .button
to not show the upper part of the barcode results sheet at the bottom of the screen and display a button that calls up the sheet instead.
💡 You can change the entire RTU UI’s color scheme using the palette feature.
Once you’ve made your changes, build and run your app.
Step 3: Scanning some barcodes!
Now you can go ahead and scan 1D and 2D barcodes – one after the other, many at the same time, and even with an AR overlay that lets you preview their values!
If you’re in need of some barcodes, we’ve got you covered:
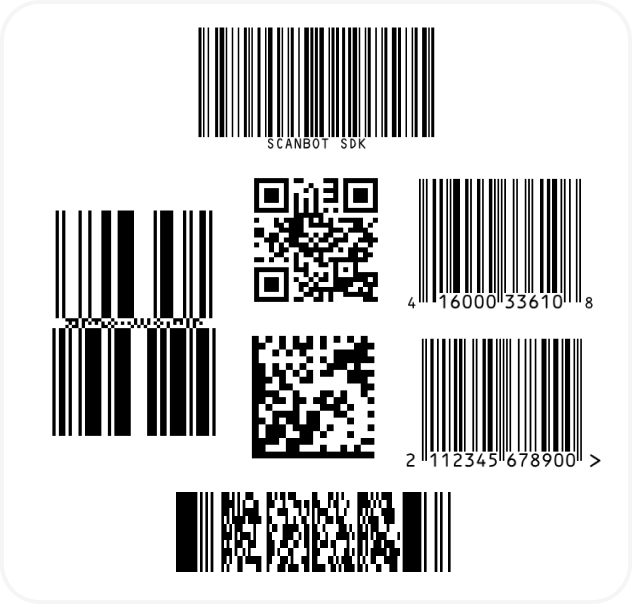
If this tutorial has piqued your interest in integrating barcode scanning functionalities into your iOS app, make sure to take a look at our SDK’s other features in our documentation.
Happy coding!