The Scanbot SDK works completely offline and never connects to the internet. This ensures that no third party can gain access to the data you scan and also allows you to use the scanner in locations without internet access.
However, this also means that the license key for your app cannot be updated automatically. Instead, you need to change it in the app binaries, which usually means publishing a new version of the app to Google Play or the App Store.
To solve this and help streamline the process, you can store the license key on your remote server. Or you can get it via a Firebase Remote Config property.
In this article, we’ll explore the latter option since many developers have Firebase Analytics or Remote Config already integrated into their applications. To demonstrate how to use this technology to automatically update your license key, we’ll show you the complete integration process for our React Native Barcode Scanner SDK using the following steps:
- Set up a new Firebase project
- Prepare the Remote Config property
- Register your app in the Firebase project
- Create a new Expo project with Firebase and the Barcode Scanner SDK
- Fetch and apply the default values to the Barcode Scanner SDK
- Test the Remote Config
Requirements
- A machine running a recent version of macOS, Windows, or Linux
- A Google account registered in Firebase
- A local Expo environment
💡 Since React Native version 0.75, it’s recommended to use frameworks such as Expo to develop React Native applications. We’ll also use Expo in this example.
Of course, you can also install the SDK in your React Native application without a framework by following the official React Native guide.
Step 1: Setting up a new Firebase project
Open the Firebase Console and click on Get Started with a Firebase project (or Create a project if you’ve used Firebase before).
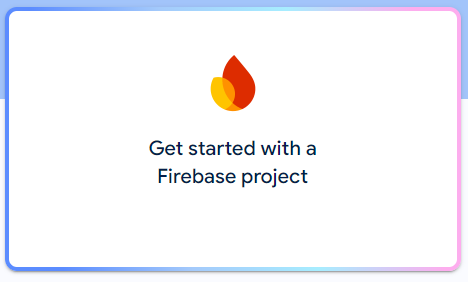
Now give the project a name:
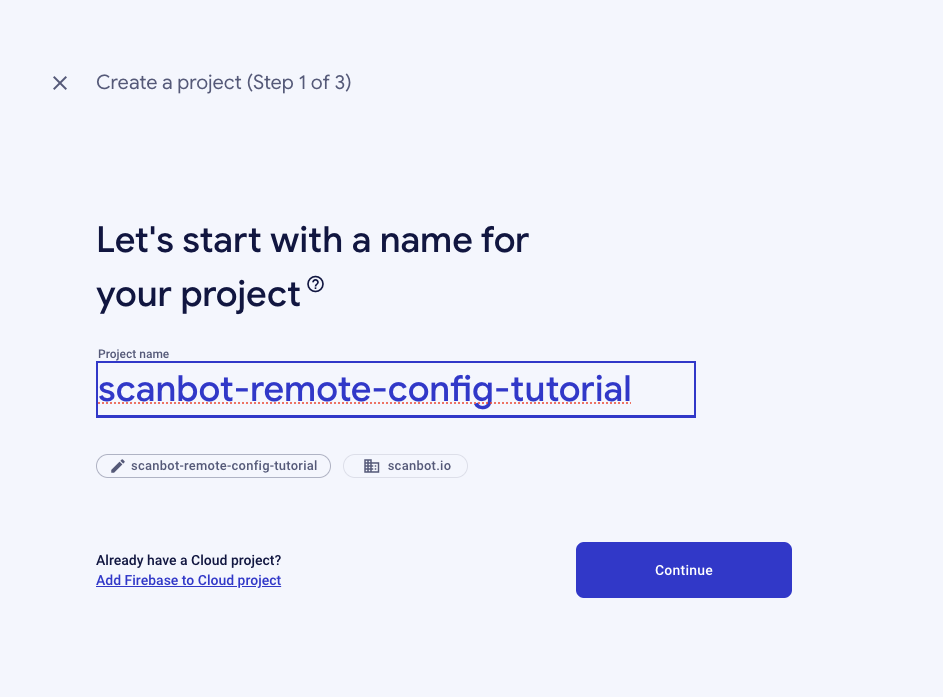
In the next step, we need to enable Google Analytics in our project because Remote Config is part of Google Analytics’ feature set.
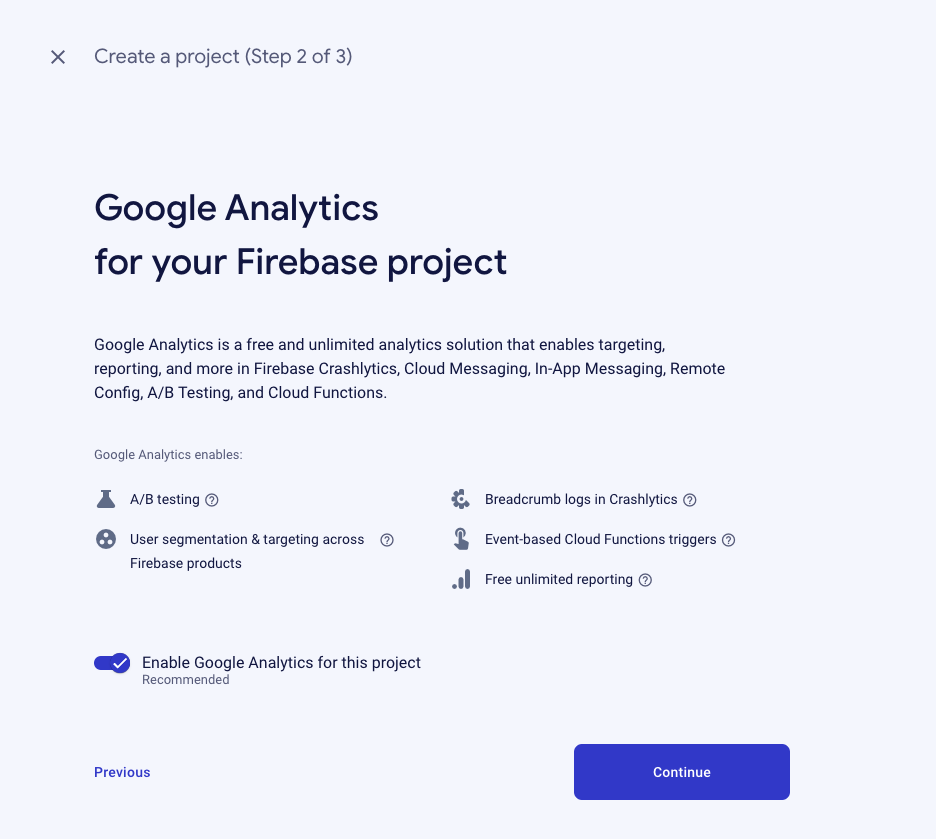
We’ll use the default selection and proceed.
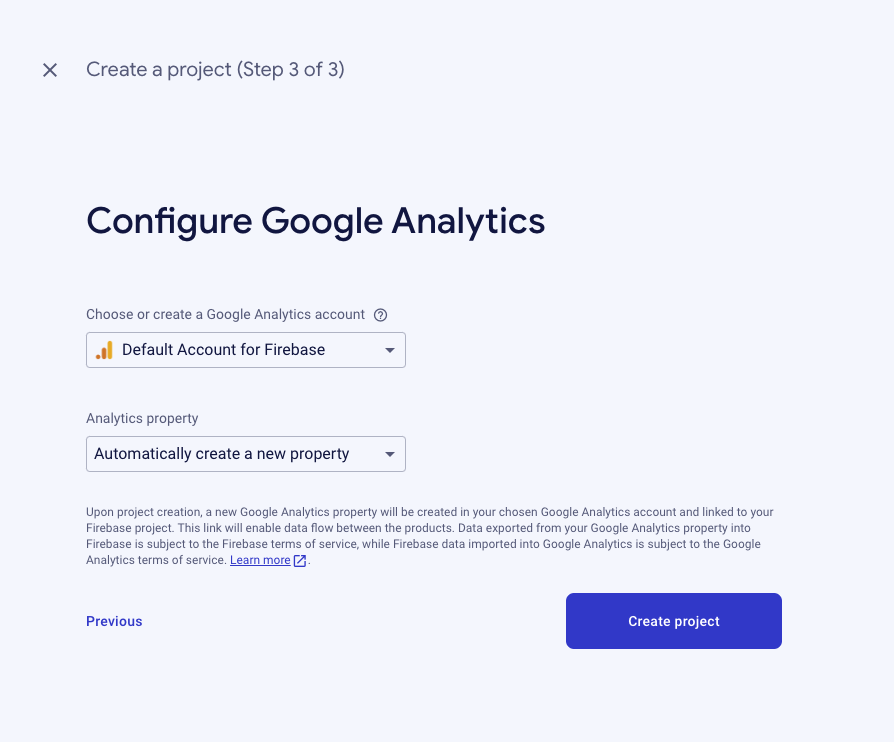
And with that, the project is ready!
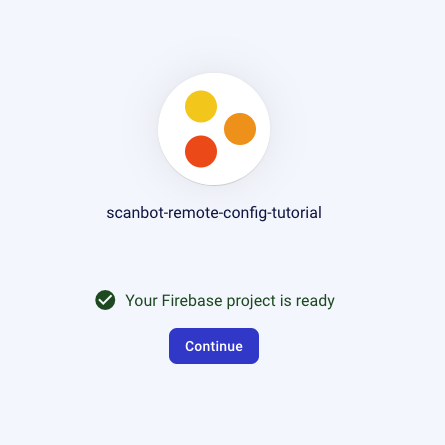
Step 2: Preparing the Remote Config property
Now we’re ready to set up the license key property in the Remote Config.
Select Run > Remote Config.
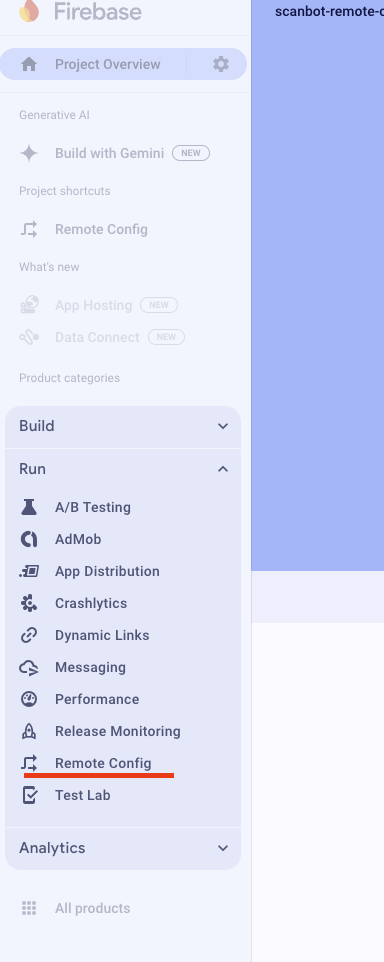
Then click on Create configuration.
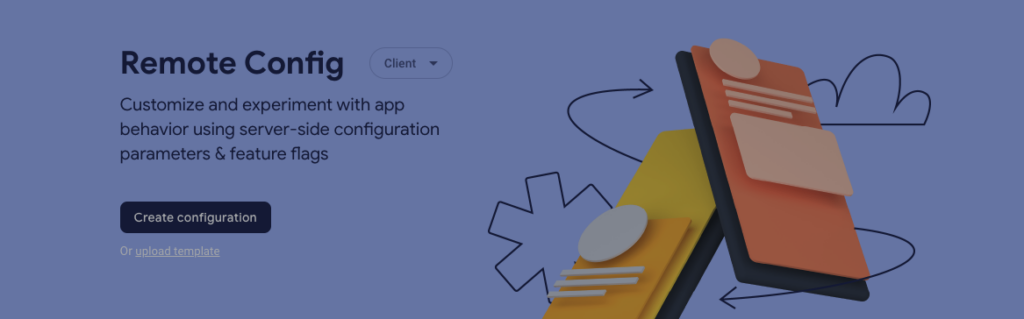
The next step is very important and involves setting up our project’s first property:
Since the Scanbot SDK’s license key contains some special characters, we can’t just use the data type String – instead, we need to switch to JSON.
Also, remember the property name used here. We’ll use scanbot_license_key for this guide.
Next, click on the double-ended arrow to set up the default value.
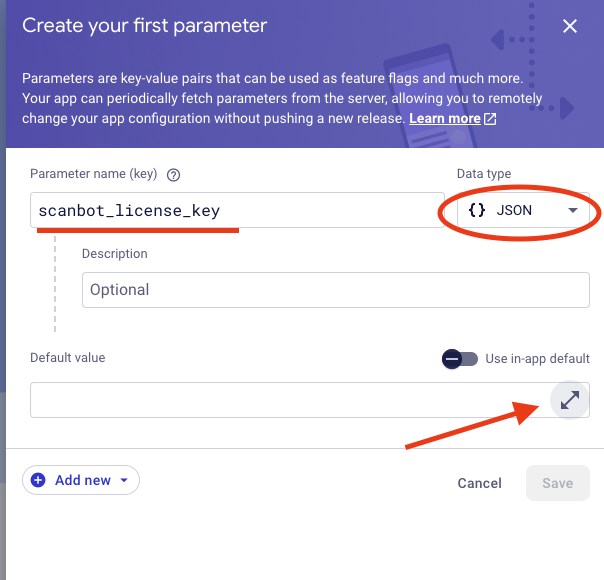
Now we need to enter the license key in a JSON format.
You can use the following JSON as a property, but don’t forget to replace <ENTER_YOUR_LICENSE_KEY_HERE>
with your actual license key. If you aren’t a customer yet and need a trial license key for your project, you can get one here.
{
"license_key": "<ENTER_YOUR_LICENSE_KEY_HERE>"
}
Now you just need to hit Save.
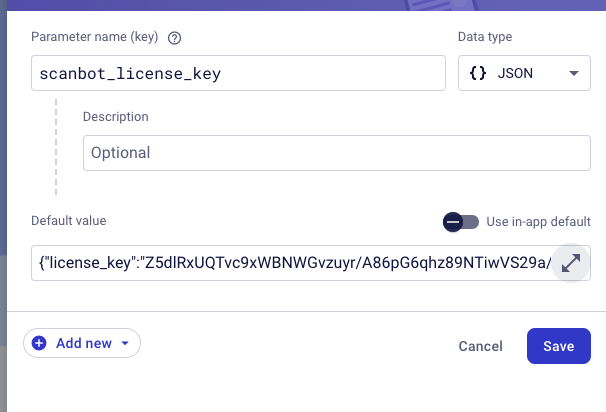
Firebase Console will remind you that you need to publish the changes. Let’s do that, so we can use the property in our app.
Step 3: Registering our app in the Firebase project
Now you’ll see the Firebase Console for your newly created project. Let’s add our Android and iOS app to it.
Android
First click on the Android icon below the project name.
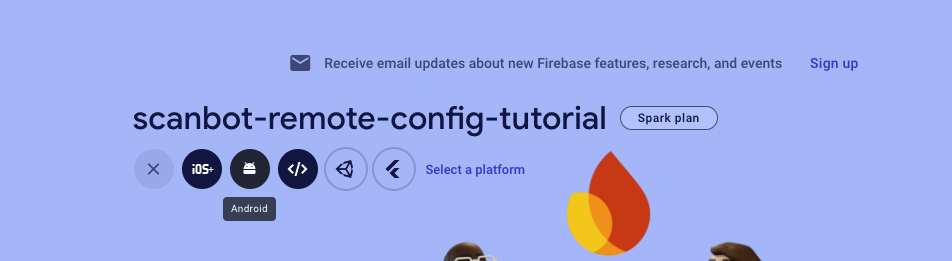
We need to specify the application ID. In a real-life scenario, enter your own project’s application ID.
For the purposes of this guide, we’ll use io.scanbot.example.remoteconfig.
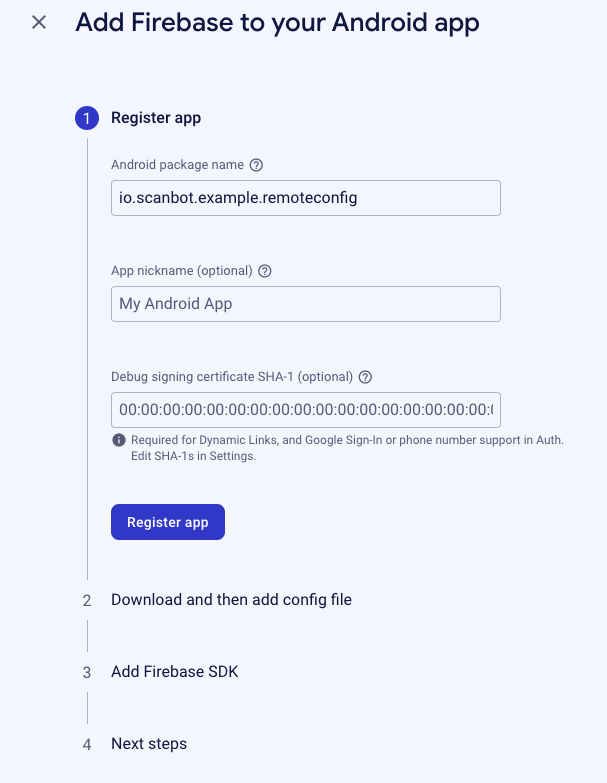
After clicking on Register app, we can download a configuration file that Firebase prepared for us by clicking on Download google-services.json.
Afterward, click on Next.
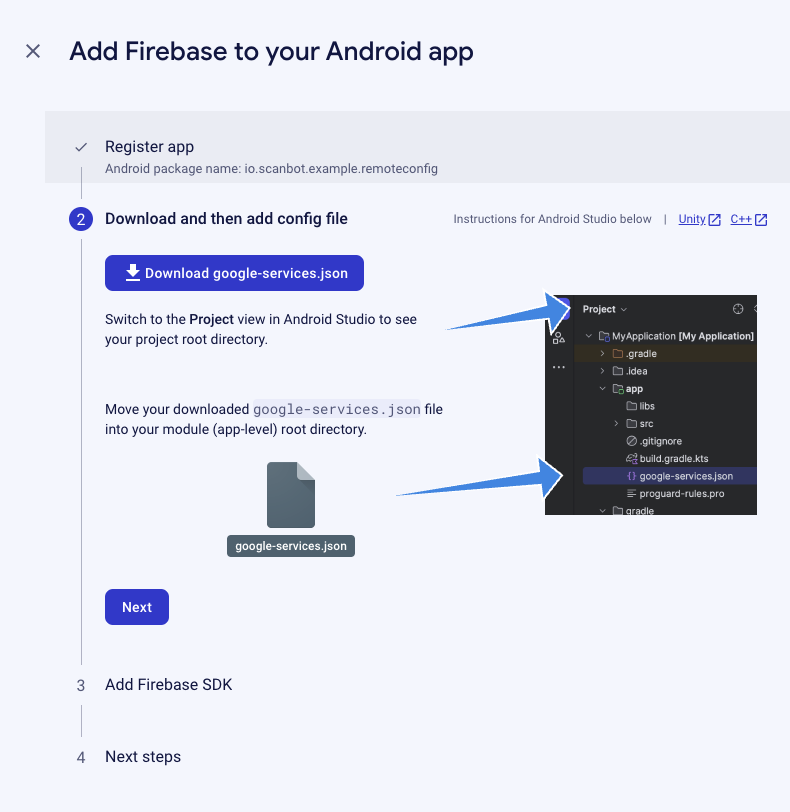
And we’re all set! Click on Continue to console.
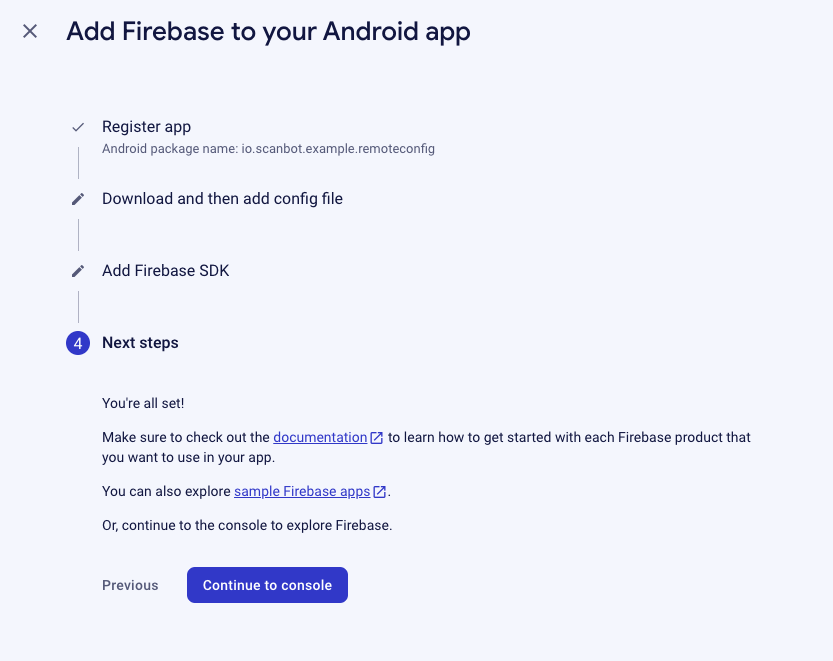
iOS
The steps for setting up iOS are similar. We start by clicking the iOS icon below the project name.
We need to specify the bundle ID. In a real-life scenario, enter your own project’s bundle ID. For the purposes of this guide, we’ll use the same ID as Android io.scanbot.example.remoteconfig.
After clicking on Register app, we can download a configuration file that Firebase prepared for us by clicking on Download GoogleService-Info.plist.
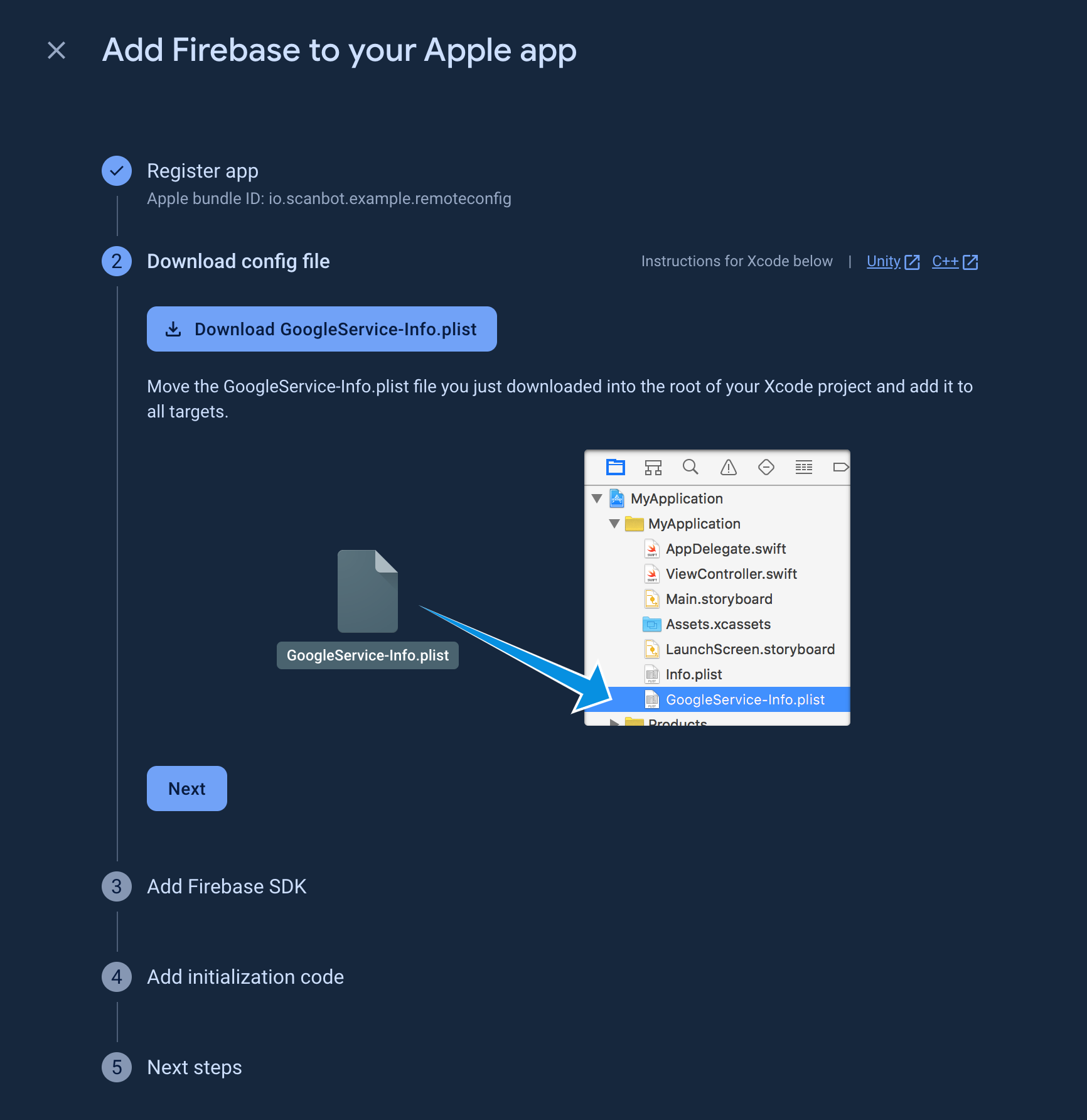
Afterward, click on Next.
And we’re all set! Click on Continue to console.
Step 4: Creating a new Expo project with Firebase and the Barcode Scanner SDK
First, let’s create our Expo app using Expo CLI, which will walk us through the setup process. To create an Expo app, run the following command in the terminal:
npx create-expo-app@latest
Let’s open the project with your favorite code editor and prepare it to install Scanbot SDK.
Remove boilerplate code
The Expo CLI has generated some screens to ensure the app isn’t empty. The generated code can be quickly removed by running the following command:
npm run reset-project
Now the app directory only contains an index file, which is our only screen, and a _layout.tsx file.
Generate the native projects
To install expo-dev-client
we need to run the following command:
npx expo install expo-dev-client
Afterwards, we can generate our iOS and Android projects with:
npx expo prebuild
Add Firebase to your project
Now we’re ready to set up Firebase in our project. The complete guide for adding Firebase to your Expo project can be found here.
First, let’s put the file we downloaded earlier (google-services.json and GoogleService-Info.plist) into the firebase folder, or anywhere you find convenient in your project (the root project directory also works).
Now we need to install the Firebase packages:
npx expo install @react-native-firebase/app @react-native-firebase/remote-config expo-build-properties
After which we need to update our Expo app.json:
{
"expo": {
"android": {
"googleServicesFile": "./firebase/google-services.json",
"package": "io.scanbot.example.remoteconfig"
},
"ios": {
"googleServicesFile": "./firebase/GoogleService-Info.plist",
"bundleIdentifier": "io.scanbot.example.remoteconfig"
},
"plugins": [
"@react-native-firebase/app",
[
"expo-build-properties",
{
"ios": {
"useFrameworks": "static"
}
}
]
]
}
}
Installing the Barcode Scanner SDK
Let’s install and configure the React Native Barcode Scanner SDK. Firstly we need to run:
npx expo install react-native-scanbot-barcode-scanner-sdk
Now that the npm package has been installed, all that’s left is to make the necessary changes to the native projects.
You can use our config plugin or manually configure the native projects. We’ll showcase both so you can pick the method you prefer.
Method A: Expo config plugin
To utilize the plugin, add the following to your app.json file:
"plugins": [
"expo-router",
[
"react-native-scanbot-barcode-scanner-sdk",
{
"iOSCameraUsageDescription": "Camera permission is needed to scan barcodes",
"androidCameraPermission": true,
"androidCameraFeature": true,
"mavenURLs": true
}
]
],
Then run:
npx expo prebuild
You can learn more about our Expo config here. You can skip the Android and iOS native changes when using this plugin.
Method B: Manual configuration
Alternatively, you can also apply the changes to the native projects manually.
Android
For Android, we first need to add the camera permissions in android/app/src/main/AndroidManifest.xml:
<uses-permission android:name="android.permission.CAMERA" />
<uses-feature android:name="android.hardware.camera" />
For development builds, we also need to add our Maven package URLs in android/build.gradle:
allprojects {
repositories {
... other maven rpositories
maven { url "https://nexus.scanbot.io/nexus/content/repositories/releases/" }
maven { url "https://nexus.scanbot.io/nexus/content/repositories/snapshots/" }
}
}
iOS
For iOS, we need to include a description for the camera permission in ios/{projectName}/Info.plist anywhere inside the element:
<key>NSCameraUsageDescription</key>
<string>Camera permission is needed to scan barcodes</string>
Step 6: Fetch and apply the default values to the Barcode Scanner SDK
All that’s left is to initialize the React Native Barcode Scanner SDK with the license key from the Firebase Remote Config.
In our _layout.ts file, we can implement the initialization like this:
useEffect(() => {
async function initializeScanbotSDKWithFirebase() {
try {
const firebaseRemoteConfig = remoteConfig()
/** Here we specify that the Remote Config shouldn’t be requested any more often than once per hour (3600 seconds) */
await firebaseRemoteConfig.setConfigSettings({
minimumFetchIntervalMillis: 3600
})
/**
* The app requests the update of the Firebase Remote Config and activates it
* When we access the value from Remote Config, we will get the value received from the server.
*/
await firebaseRemoteConfig.fetchAndActivate()
/**
* Here we access the Remote Config configuration and take the string value from the JSON.
* Then we pass this string to `ScanbotBarcodeSDK.initializeSdk`.
*/
const licenceKeyObject = firebaseRemoteConfig.getString("scanbot_license_key")
const licenseKey = JSON.parse(licenceKeyObject)['license_key']
const licenseMessage = await ScanbotBarcodeSDK.initializeSdk({licenseKey: licenseKey})
console.log(licenseMessage);
} catch (e: any) {
console.log("An error has occurred while initializing", e.message)
}
}
initializeScanbotSDKWithFirebase()
}, []);
Now that our project is ready, we can start the app to check if the config is applied.
To run our app for Android and iOS, we can use the following commands:
For Android:
expo run:android --device
For iOS:
expo run:ios --device
Step 7: Testing the Remote Config
It’s finally time to test what happens when we actually change the value on the server. Let’s return to the Firebase Console and edit our property’s default value.
For testing purposes, we will set the value to random_value
and hit Save.
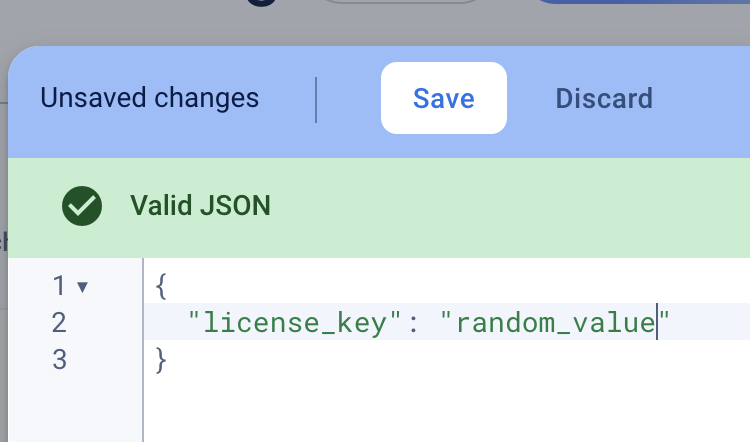
In the next dialog, click on Save again and publish the changes.
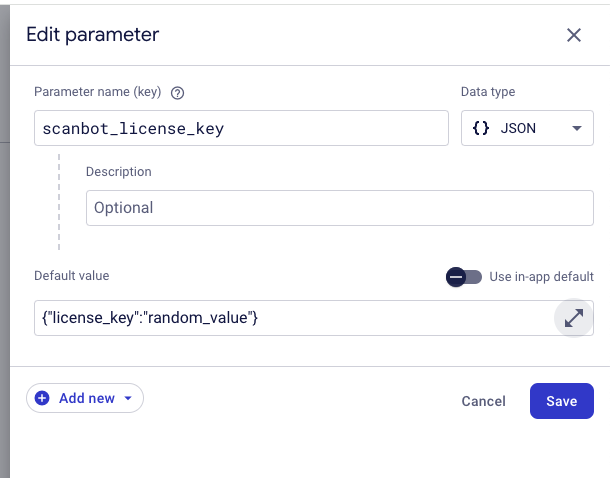
The update is now live. Since we’ve set up our app to not request updates more often than once per hour, it might take some time until the value is applied in the app. For testing purposes, we can also set minimumFetchIntervalInSeconds
to 1
.
Let’s see if the value is now applied in the app:

Yes! We see that the value from the Remote Config is now “random_value”. Since that’s not a valid license key, the Scanbot SDK reports that the license key is corrupted.
Let’s change it back to the real license key and see if the license check is working correctly again.

Great! As we can see, the license key is now coming not from the default values but from the Firebase Remote Config servers and is applied correctly. That means that from now on, we can update the license key in the app without publishing a new build to Google Play, which can help you save a lot of time and effort in the long run.
Although Firebase Remote Config is the easiest way to achieve this, it does come with some disadvantages. The biggest one is that if you use Firebase Remote Config, you have to include the whole Firebase/Google Analytics package in your app. This has implications for complying with data privacy regulations like the GDPR since this gives Google access to some meta information of your users.
An alternative solution would be to store the license key on your web server, accessing it via an API, and then storing it in Shared Preferences, though this can be a much more complicated process. That’s why many developers prefer the Firebase Remote Config method.
Conclusion
We hope this guide on how to do license checks using Firebase Remote Config was helpful to you!
If you have questions about this guide or ran into any issues, we’re happy to help! Just shoot us an email via tutorial-support@scanbot.io. And keep an eye on this blog for more guides and tutorials!