In this tutorial, you will learn how to build a web app for scanning documents and exporting them as PDFs using nothing but HTML, JavaScript, and the Scanbot Web Document Scanner SDK.
The app will initially display a page with a “Start scanning” button and an “Export PDF” button. Tapping on “Start scanning” opens the document scanner interface, enabling the user to scan one or several pages of a document. After tapping on “Submit”, the user will be transported back to the initial page and can choose to download a PDF file of the scanned document by tapping on the “Export PDF” button.
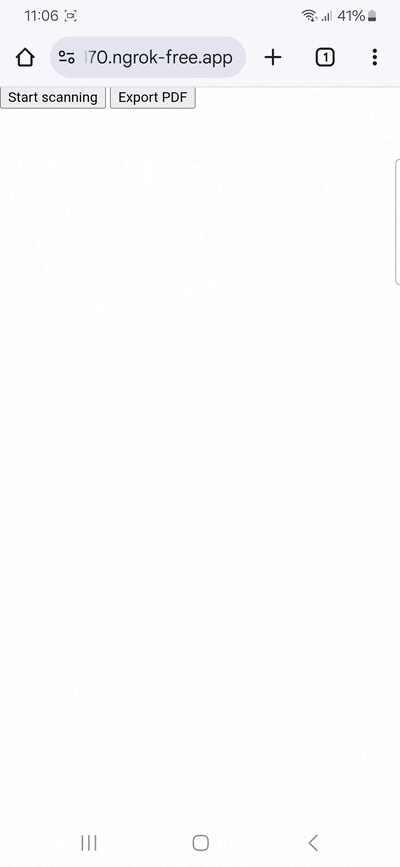
To accomplish this, we’ll follow the steps below:
- Downloading the SDK files
- Creating the HTML page and configuring the Web SDK
- Implementing the PDF export
Let’s get started!
Want to see the final code right away? Click here.
index.html:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=0" />
<title>My Scanner App</title>
</head>
<body style="margin: 0">
<button id="start-scanning">Start scanning</button>
<button id="export-pdf">Export PDF</button>
<script type="module">
import "./scanbot-web-sdk/bundle/ScanbotSDK.ui2.min.js";
const sdk = await ScanbotSDK.initialize({
enginePath: "scanbot-web-sdk/bundle/bin/complete/"
});
let scanResult;
document.getElementById("start-scanning").addEventListener("click", async () => {
const config = new ScanbotSDK.UI.Config.DocumentScanningFlow();
scanResult = await ScanbotSDK.UI.createDocumentScanner(config);
});
document.getElementById("export-pdf").addEventListener("click", async () => {
const pages = scanResult?.document?.pages;
if (!pages || !pages.length) {
alert("Please scan a document first.");
return;
}
const options = { pageSize: "A4", pageDirection: "PORTRAIT", pageFit: "FIT_IN", dpi: 72, jpegQuality: 80 };
const bytes = await scanResult?.document?.createPdf(options);
function saveBytes(data, name) {
const extension = name.split(".")[1];
const a = document.createElement("a");
document.body.appendChild(a);
a.style = "display: none";
const blob = new Blob([data], {type: `application/${extension}`});
const url = window.URL.createObjectURL(blob);
a.href = url;
a.download = name;
a.click();
window.URL.revokeObjectURL(url);
}
saveBytes(bytes, "generated.pdf");
});
</script>
</body>
</html>
Requirements
The Scanbot Web SDK is optimized for mobile devices. Although you can test the document scanner app’s basic functionality using your laptop camera or a web cam, we recommend you run it on a smartphone.
You can use a service like ngrok, which creates a tunnel to one of their SSL-certified domains. Their Quick Start guide will help you get up and running quickly.
Step 1: Download the SDK
First, create a new empty directory for your app and name it, e.g. “document-scanner-app”.
Then download the Scanbot SDK package directly from the npm registry.
💡 You can also use npm to download and install the package, but in this tutorial, we’ll do it manually. You can check the latest SDK version in the changelog.
Unzip the downloaded files into document-scanner-app. If the unzipped folder is named “package” or similar, rename it to “scanbot-web-sdk” or make sure to later provide the correct directory path in the code.
Your folder structure should look like this:
document-scanner-app/
|- scanbot-web-sdk/
|- webpack/
|- bundle/
|- @types/
|- index.js
|- ui.js
... (and some other files)
Step 2: Create the HTML page and configure the Web SDK
To create the HTML with the Scanbot SDK, first create an index.html file in the document-scanner-app folder. Then add the following code to the file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<!-- We prevent the user from zooming on mobile device -->
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=0" />
<title>My Scanner App</title>
</head>
<body style="margin: 0">
<button id="start-scanning">Start scanning</button>
<script type="module">
// We import the necessary ScanbotSDK module
import "./scanbot-web-sdk/bundle/ScanbotSDK.ui2.min.js";
// When initializing the SDK, we specify the path to the document scanner engine
const sdk = await ScanbotSDK.initialize({
enginePath: "scanbot-web-sdk/bundle/bin/complete/"
});
document.getElementById("start-scanning").addEventListener("click", async () => {
// We create a new default configuration for the document scanner
const config = new ScanbotSDK.UI.Config.DocumentScanningFlow();
// We create a document scanner UI component
const scanResult = await ScanbotSDK.UI.createDocumentScanner(config);
return scanResult;
});
</script>
</body>
</html>
The code block above:
- Adds the “Start scanning” button.
- Imports and initializes the Scanbot SDK.
- Executes the document scanner interface from the Scanbot SDK when the user taps the “Start scanning” button.
💡 Without a license key, our SDK only runs for 60 seconds per session. This is more than enough for the purposes of our tutorial, but if you like, you can generate a license key on our website.
Feel free to run the app to see if everything is working so far.
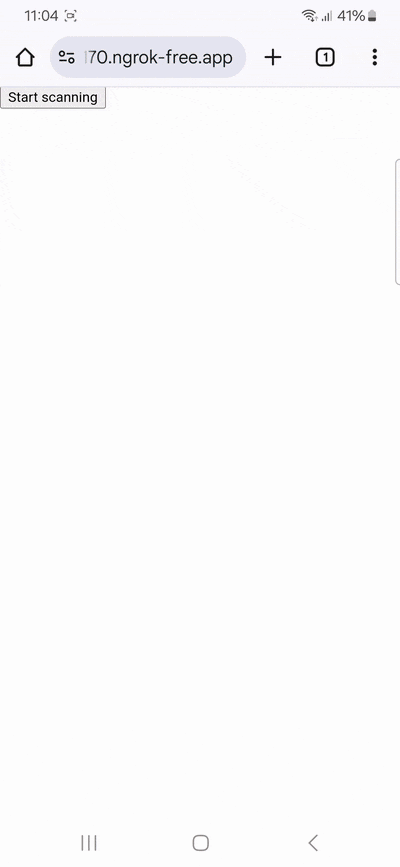
At the moment, the user cannot do anything with the scanned pages, but we’ll change that in the next step.
Step 3: Implement the PDF export
To allow users to export their scanned documents as PDF files, we need to do the following:
Add a button to our start screen that starts the PDF generation when clicked.
<button id="export-pdf">Export PDF</button>
...
document.getElementById("export-pdf").addEventListener("click", async () => {});
Make sure there’s a document to export.
const pages = scanResult?.document?.pages;
if (!pages || !pages.length) {
alert("Please scan a document first.");
return;
}
Configure how the PDF should be generated. You can adjust this as you see fit.
const options = { pageSize: "A4", pageDirection: "PORTRAIT", pageFit: "FIT_IN", dpi: 72, jpegQuality: 80 };
Use the SDK’s built-in createPdf()
method to generate the PDF.
const bytes = await scanResult?.document?.createPdf(options);
Save the PDF and trigger the download.
function saveBytes(data, name) {
const extension = name.split(".")[1];
const a = document.createElement("a");
document.body.appendChild(a);
a.style = "display: none";
const blob = new Blob([data], {type: `application/${extension}`});
const url = window.URL.createObjectURL(blob);
a.href = url;
a.download = name;
a.click();
window.URL.revokeObjectURL(url);
}
saveBytes(bytes, "generated.pdf");
Your index.html should now look something like this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=0" />
<title>My Scanner App</title>
</head>
<body style="margin: 0">
<button id="start-scanning">Start scanning</button>
<button id="export-pdf">Export PDF</button>
<script type="module">
import "./scanbot-web-sdk/bundle/ScanbotSDK.ui2.min.js";
const sdk = await ScanbotSDK.initialize({
enginePath: "scanbot-web-sdk/bundle/bin/complete/"
});
let scanResult;
document.getElementById("start-scanning").addEventListener("click", async () => {
const config = new ScanbotSDK.UI.Config.DocumentScanningFlow();
scanResult = await ScanbotSDK.UI.createDocumentScanner(config);
});
document.getElementById("export-pdf").addEventListener("click", async () => {
const pages = scanResult?.document?.pages;
if (!pages || !pages.length) {
alert("Please scan a document first.");
return;
}
const options = { pageSize: "A4", pageDirection: "PORTRAIT", pageFit: "FIT_IN", dpi: 72, jpegQuality: 80 };
const bytes = await scanResult?.document?.createPdf(options);
function saveBytes(data, name) {
const extension = name.split(".")[1];
const a = document.createElement("a");
document.body.appendChild(a);
a.style = "display: none";
const blob = new Blob([data], {type: `application/${extension}`});
const url = window.URL.createObjectURL(blob);
a.href = url;
a.download = name;
a.click();
window.URL.revokeObjectURL(url);
}
saveBytes(bytes, "generated.pdf");
});
</script>
</body>
</html>
Now run your app to test scanning a single- or multi-page document and exporting it as a PDF.
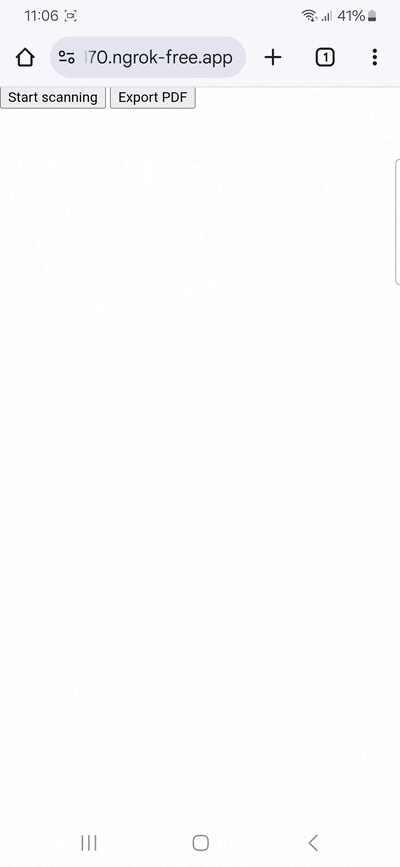
Conclusion
🎉 Congratulations! You can now scan documents from your browser and export them as PDFs!
If this tutorial has piqued your interest in integrating document scanning functionalities into your web app or website, make sure to take a look at our SDK’s other neat features in our documentation – or run our example project for a more hands-on experience.
Should you have questions about this tutorial or run into any issues, we’re happy to help! Just shoot us an email via tutorial-support@scanbot.io.
Happy scanning! 🤳