In this tutorial, we’ll use Android Studio and the Scanbot SDK Android Barcode Scanner library to create a simple app offering three different scanning modes: scanning one barcode at a time, multiple barcodes at once, and displaying the barcode values in the viewfinder using the SDK’s AR overlay.
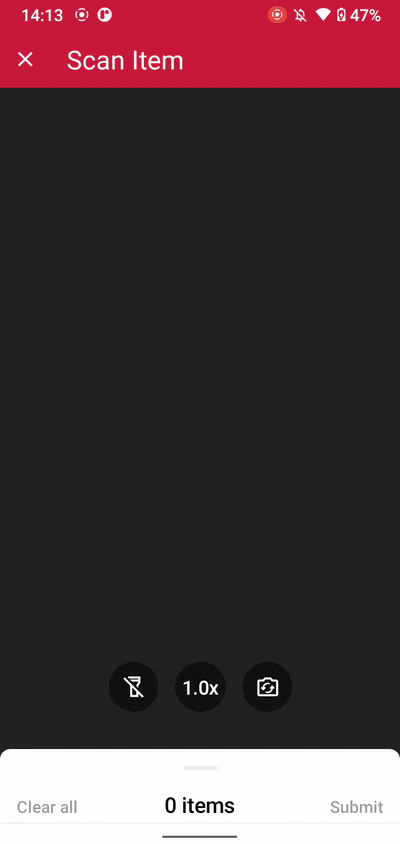
We’ll achieve this in five steps:
- Prepare the project
- Initialize the SDK
- Set up the main screen
- Implement the scanning modes
- Scan some barcodes!
All you need is the latest version of Android Studio and you’re good to go.
How to integrate our Android Barcode Scanner library in 5 steps
Step 1: Preparing the project
Create a new Empty View Activity and name the project (e.g., “Android Barcode Scanner”).
When your project is ready, go to settings.gradle.kts and add the Maven repositories for our SDK:
dependencyResolutionManagement {
repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS)
repositories {
google()
mavenCentral()
// Add the repositories here:
maven(url = "https://nexus.scanbot.io/nexus/content/repositories/releases/")
maven(url = "https://nexus.scanbot.io/nexus/content/repositories/snapshots/")
}
}
Now go to app/build.gradle.kts and add the dependencies for the Scanbot SDK and the RTU UI:
dependencies {
implementation("io.scanbot:scanbot-barcode-scanner-sdk:5.2.0")
implementation("io.scanbot:rtu-ui-v2-barcode:5.2.0")
Sync the project.
💡 We use Barcode Scanner SDK version 5.2.0 in this tutorial. You can find the latest version in the changelog.
Since we need to access the device camera to scan barcodes, let’s also add the necessary permissions in AndroidManifest.xml:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.CAMERA" />
<uses-feature android:name="android.hardware.camera" />
<application
...
Step 2: Initializing the SDK
Before we can use the Scanbot Barcode Scanner SDK, we need to initialize it. The recommended approach is to do it in your Application
implementation. This ensures the SDK is correctly initialized even when the app’s process is restored after being terminated in the background.
First, we need to create an Application
subclass by right-clicking on the folder app/kotlin+java/com.example.androidbarcodescanner, selecting New > Kotlin Class/File, and naming it (e.g. “ExampleApplication”).
In the resulting ExampleApplication.kt, let’s first add the necessary imports:
import android.app.Application
import io.scanbot.sdk.barcode_scanner.ScanbotBarcodeScannerSDKInitializer
Then we make ExampleApplication
extend the Application
class by adding : Application()
and add the code for initializing the SDK inside it:
class ExampleApplication : Application() {
override fun onCreate() {
super.onCreate()
ScanbotBarcodeScannerSDKInitializer()
// Optional: uncomment the next line if you have a license key.
// .license(this, LICENSE_KEY)
.initialize(this)
}
}
💡 Without a license key, our SDK only runs for 60 seconds per session. This is more than enough for the purposes of our tutorial, but if you like, you can generate a license key. Just make sure to change your applicationId
in app/build.gradle.kts to io.scanbot.androidbarcodescanner
and use that ID for generating the license.
Finally, we need to register the Example Application
class in AndroidManifest.xml:
<application
android:name=".ExampleApplication"
...
Step 3: Setting up the main screen
We’re going to build a rudimentary UI so we can quickly access the different scanning modes in our finished app.
For this tutorial, we’re going to go with this simple three-button layout, which you can copy into app/res/layout/activity_main.xml:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/btn_single_scanning"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="Single-barcode scanning"
app:layout_constraintBottom_toTopOf="@id/btn_multi_scanning"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
android:layout_marginBottom="20dp" />
<Button
android:id="@+id/btn_multi_scanning"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="Multi-barcode scanning"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
android:layout_marginTop="20dp"
android:layout_marginBottom="20dp" />
<Button
android:id="@+id/btn_ar_overlay"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="AR overlay"
app:layout_constraintTop_toBottomOf="@id/btn_multi_scanning"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
android:layout_marginTop="20dp" />
</androidx.constraintlayout.widget.ConstraintLayout>
Step 4: Implementing the scanning modes
Now we’ll connect each button with one of our RTU UI’s scanning modes, starting with single-barcode scanning.
Go to MainActivity.kt and add the necessary imports:
import android.widget.Button
import android.widget.Toast
import androidx.activity.result.ActivityResultLauncher
import io.scanbot.sdk.ui_v2.barcode.BarcodeScannerActivity
import io.scanbot.sdk.ui_v2.common.activity.registerForActivityResultOk
import io.scanbot.sdk.ui_v2.barcode.common.mappers.COMMON_CODES
import io.scanbot.sdk.ui_v2.barcode.configuration.BarcodeFormat
import io.scanbot.sdk.ui_v2.barcode.configuration.CollapsedVisibleHeight
import io.scanbot.sdk.ui_v2.barcode.configuration.BarcodeScannerConfiguration
import io.scanbot.sdk.ui_v2.barcode.configuration.MultipleBarcodesScanningMode
import io.scanbot.sdk.ui_v2.barcode.configuration.MultipleScanningMode
import io.scanbot.sdk.ui_v2.barcode.configuration.SheetMode
import io.scanbot.sdk.ui_v2.barcode.configuration.SingleScanningMode
import io.scanbot.sdk.ui_v2.common.ScanbotColor
With that out of the way, let’s implement the first scanning mode.
Single-barcode scanning
In the MainActivity
class, add a private property that we’ll use to launch the barcode scanner.
private val barcodeResultLauncher: ActivityResultLauncher<BarcodeScannerConfiguration> =
registerForActivityResultOk(BarcodeScannerActivity.ResultContract()) { resultEntity ->
// Barcode Scanner result callback:
// Get the first scanned barcode from the result object...
val barcodeItem = resultEntity.result?.items?.first()
// ... and process the result as needed. For example, display as a Toast:
Toast.makeText(
this,
"Scanned: ${barcodeItem?.text} (${barcodeItem?.type})",
Toast.LENGTH_LONG
).show()
}
Next, in the onCreate()
method, we set up an OnClickListener
for our Single-barcode scanning button. When the button is clicked, it should launch barcodeResultLauncher
using a configuration object we’ll set up in the next step:
findViewById<Button>(R.id.btn_single_scanning).setOnClickListener {
barcodeResultLauncher.launch(config)
}
Now paste the configuration for the RTU UI’s single-barcode scanning mode (from the documentation) above barcodeResultLauncher.launch(config)
:
findViewById<Button>(R.id.btn_single_scanning).setOnClickListener {
val config = BarcodeScannerConfiguration().apply {
// Configure parameters (use explicit `this.` receiver for better code completion):
// Initialize the use case for single scanning.
this.useCase = SingleScanningMode().apply {
// Enable and configure the confirmation sheet.
this.confirmationSheetEnabled = true
this.sheetColor = ScanbotColor("#FFFFFF")
// Hide/unhide the barcode image.
this.barcodeImageVisible = true
// Configure the barcode title of the confirmation sheet.
this.barcodeTitle.visible = true
this.barcodeTitle.color = ScanbotColor("#000000")
// Configure the barcode subtitle of the confirmation sheet.
this.barcodeSubtitle.visible = true
this.barcodeSubtitle.color = ScanbotColor("#000000")
// Configure the cancel button of the confirmation sheet.
this.cancelButton.text = "Close"
this.cancelButton.foreground.color = ScanbotColor("#C8193C")
this.cancelButton.background.fillColor = ScanbotColor("#00000000")
// Configure the submit button of the confirmation sheet.
this.submitButton.text = "Submit"
this.submitButton.foreground.color = ScanbotColor("#FFFFFF")
this.submitButton.background.fillColor = ScanbotColor("#C8193C")
// Configure other parameters, pertaining to single-scanning mode as needed.
}
// Set an array of accepted barcode types.
this.recognizerConfiguration.barcodeFormats = BarcodeFormat.COMMON_CODES
// Configure other parameters as needed.
}
barcodeResultLauncher.launch(config)
}
If you want, you can run the app to try out the single-barcode scanning feature before we move on to the next mode.
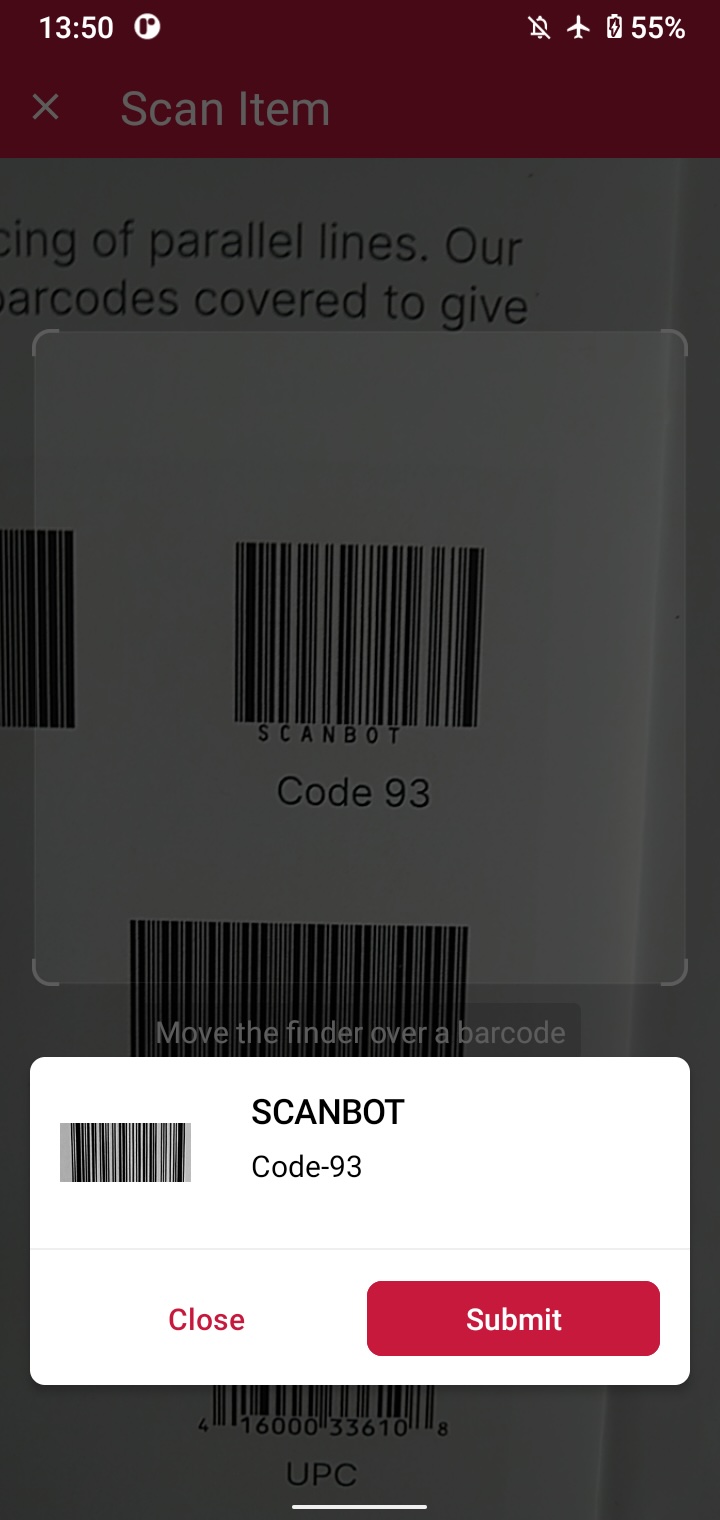
Multi-barcode scanning
Set up another OnClickListener
, this time for the Multi-barcode scanning button …
findViewById<Button>(R.id.btn_multi_scanning).setOnClickListener {
barcodeResultLauncher.launch(config)
}
… and paste in the configuration for multi-scanning above barcodeResultLauncher.launch(config)
:
findViewById<Button>(R.id.btn_multi_scanning).setOnClickListener {
val config = BarcodeScannerConfiguration().apply {
// Configure parameters (use explicit `this.` receiver for better code completion):
// Initialize the use case for multiple scanning.
this.useCase = MultipleScanningMode().apply {
// Set the counting mode.
this.mode = MultipleBarcodesScanningMode.COUNTING
// Set the sheet mode for the barcodes preview.
this.sheet.mode = SheetMode.COLLAPSED_SHEET
// Set the height for the collapsed sheet.
this.sheet.collapsedVisibleHeight = CollapsedVisibleHeight.LARGE
// Enable manual count change.
this.sheetContent.manualCountChangeEnabled = true
// Set the delay before same barcode counting repeat.
this.countingRepeatDelay = 1000
// Configure the submit button.
this.sheetContent.submitButton.text = "Submit"
this.sheetContent.submitButton.foreground.color = ScanbotColor("#000000")
// Configure other parameters, pertaining to multiple-scanning mode as needed.
}
// Set an array of accepted barcode types.
this.recognizerConfiguration.barcodeFormats = BarcodeFormat.COMMON_CODES
// Configure other parameters as needed.
}
barcodeResultLauncher.launch(config)
}
Try it out if you like and then move on to the final scanning mode.
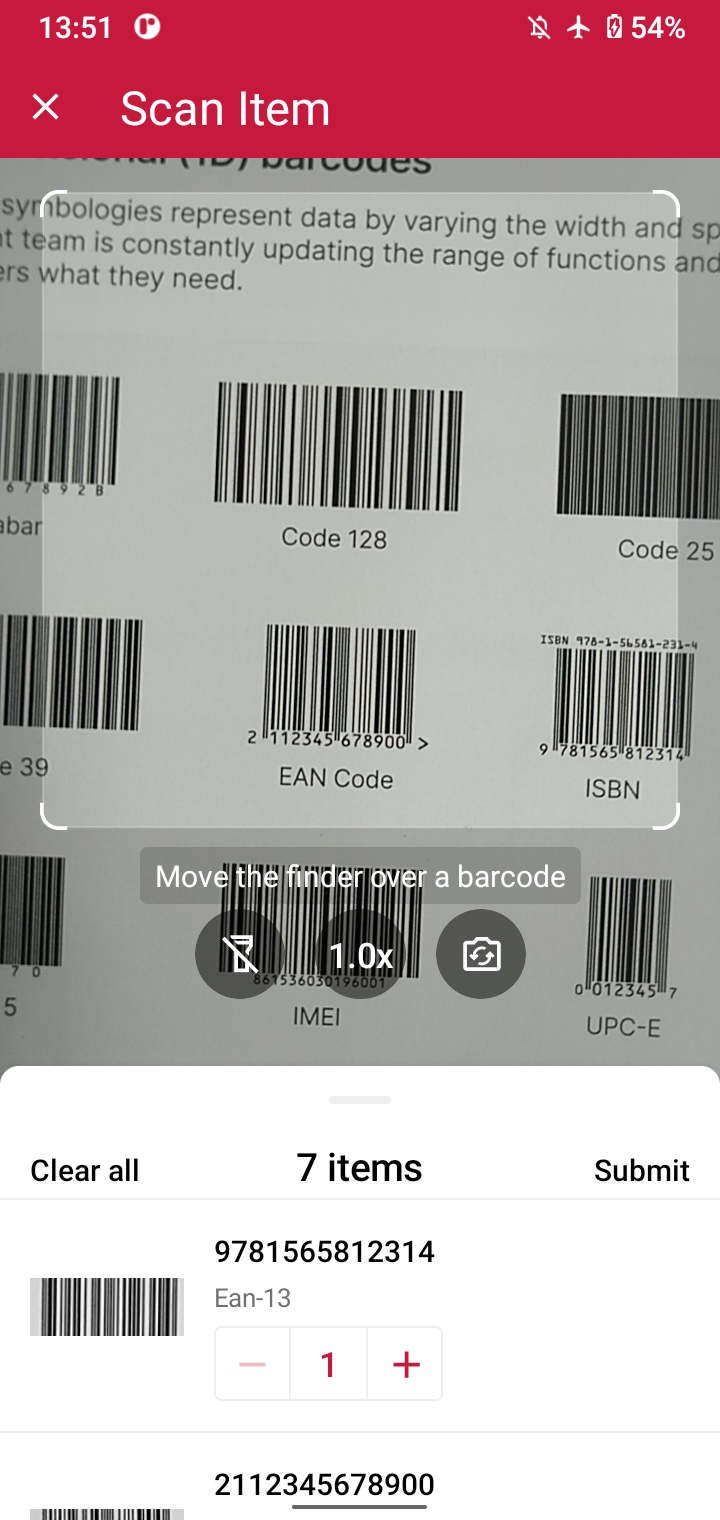
AR overlay
You know the drill. Set up an OnClickListener
…
findViewById<Button>(R.id.btn_ar_overlay).setOnClickListener {
barcodeResultLauncher.launch(config)
}
… and paste in the configuration for the AR overlay:
findViewById<Button>(R.id.btn_ar_overlay).setOnClickListener {
findViewById<Button>(R.id.btn_ar_overlay).setOnClickListener {
val config = BarcodeScannerConfiguration().apply {
// Configure parameters (use explicit `this.` receiver for better code completion):
// Configure the use case.
this.useCase = MultipleScanningMode().apply {
this.mode = MultipleBarcodesScanningMode.UNIQUE
this.sheet.mode = SheetMode.COLLAPSED_SHEET
this.sheet.collapsedVisibleHeight = CollapsedVisibleHeight.SMALL
// Configure AR Overlay.
this.arOverlay.visible = true
this.arOverlay.automaticSelectionEnabled = false
// Configure other parameters, pertaining to use case as needed.
}
// Set an array of accepted barcode types.
this.recognizerConfiguration.barcodeFormats = BarcodeFormat.COMMON_CODES
// Configure other parameters as needed.
}
barcodeResultLauncher.launch(config)
}
}
Build and run the app… and that’s it! 🎉
Step 5: Scanning some barcodes!
You can now scan barcodes one at a time, multiple barcodes in one go, and display their values on the screen via the AR overlay.
Happy scanning! 🤳
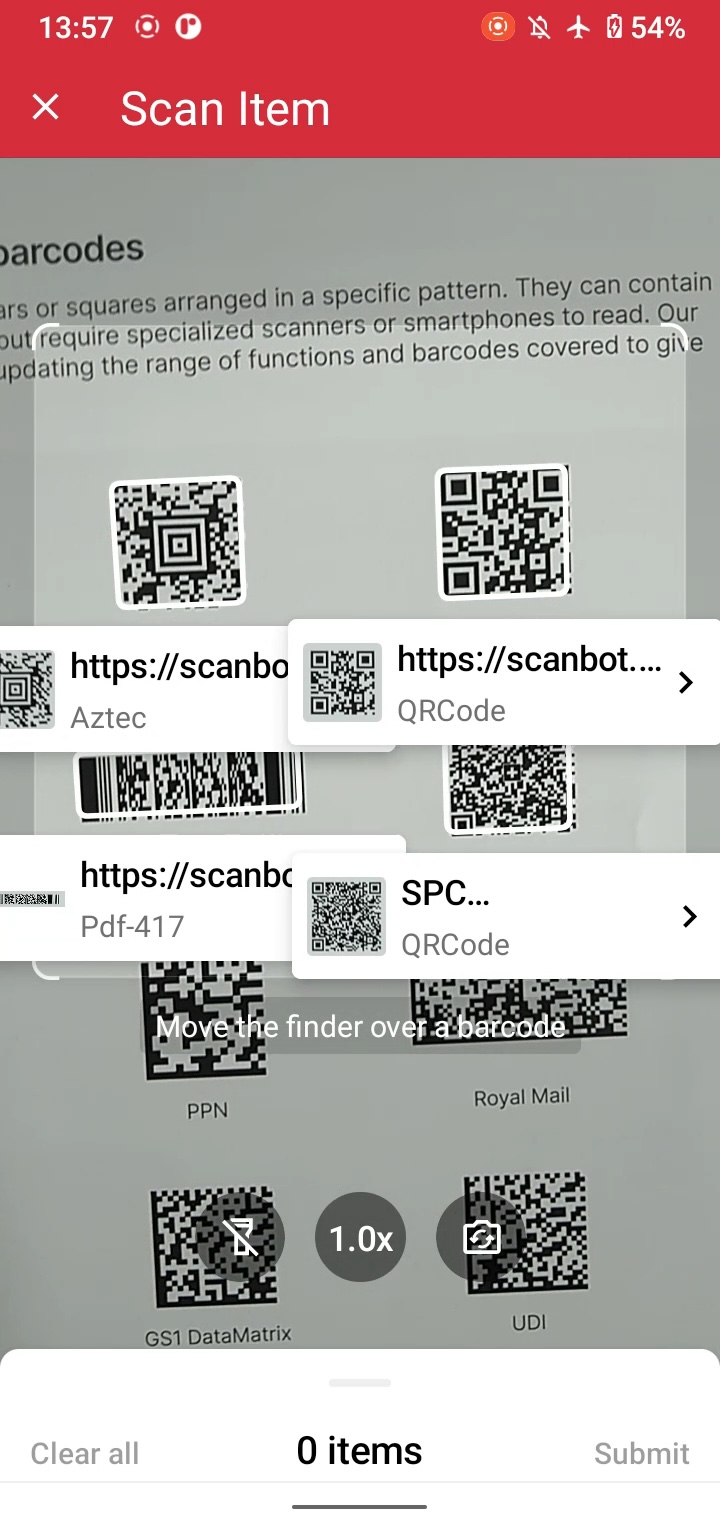
Conclusion
If this tutorial has piqued your interest in integrating barcode scanning functionalities into your Android app, make sure to take a look at our SDK’s other neat features in our documentation – or run our example project for a more hands-on experience.
Should you have questions about this tutorial or run into any issues, we’re happy to help! Just shoot us an email via tutorial-support@scanbot.io.